用两个类写代码,交我java面对对象,带注释和英语翻译
时间: 2024-05-27 21:10:41 浏览: 73
Class 1: Student
/**
* This class represents a student with a name and a grade.
*/
public class Student {
private String name;
private int grade;
/**
* Constructor for the Student class.
* @param name The name of the student.
* @param grade The grade of the student.
*/
public Student(String name, int grade) {
this.name = name;
this.grade = grade;
}
/**
* Getter method for the name of the student.
* @return The name of the student.
*/
public String getName() {
return name;
}
/**
* Setter method for the name of the student.
* @param name The new name of the student.
*/
public void setName(String name) {
this.name = name;
}
/**
* Getter method for the grade of the student.
* @return The grade of the student.
*/
public int getGrade() {
return grade;
}
/**
* Setter method for the grade of the student.
* @param grade The new grade of the student.
*/
public void setGrade(int grade) {
this.grade = grade;
}
}
Class 2: School
/**
* This class represents a school with a list of students.
*/
public class School {
private List<Student> students;
/**
* Constructor for the School class.
* Initializes an empty list of students.
*/
public School() {
students = new ArrayList<>();
}
/**
* Adds a student to the list of students in the school.
* @param student The student to add.
*/
public void addStudent(Student student) {
students.add(student);
}
/**
* Removes a student from the list of students in the school.
* @param student The student to remove.
*/
public void removeStudent(Student student) {
students.remove(student);
}
/**
* Calculates the average grade of all the students in the school.
* @return The average grade of all the students in the school.
*/
public double calculateAverageGrade() {
int totalGrade = 0;
for (Student s : students) {
totalGrade += s.getGrade();
}
return (double)totalGrade / students.size();
}
/**
* Getter method for the list of students in the school.
* @return The list of students in the school.
*/
public List<Student> getStudents() {
return students;
}
}
Explanation:
The first class, Student, represents a student with a name and a grade. It has a constructor that takes in a name and a grade and initializes the corresponding instance variables. It also has getter and setter methods for both instance variables.
The second class, School, represents a school with a list of students. It has a constructor that initializes an empty list of students. It has methods to add and remove students from the list, as well as a method to calculate the average grade of all the students in the school. It also has a getter method for the list of students.
阅读全文
相关推荐


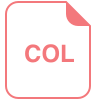
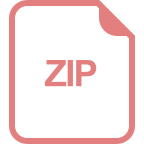
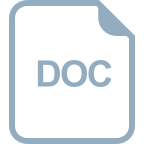
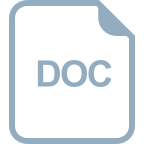
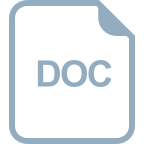
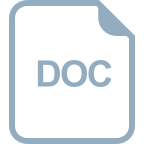
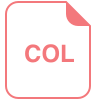
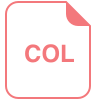
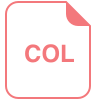
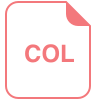
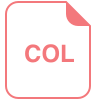
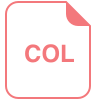
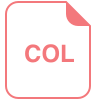
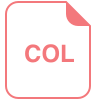