用python采集传感器数据并以msv格式输出
时间: 2023-06-12 20:06:03 浏览: 222
要采集传感器数据,你需要先连接传感器,并根据传感器的类型编写相应的代码。这里我们以DS18B20温度传感器为例,通过树莓派采集数据。
```python
import os
# 初始化DS18B20传感器
os.system('modprobe w1-gpio')
os.system('modprobe w1-therm')
# 读取传感器数据
def read_temperature():
sensor_file = '/sys/bus/w1/devices/28-00000xxxxxxx/w1_slave'
try:
with open(sensor_file, 'r') as f:
lines = f.readlines()
crc = lines[0].strip()[-3:]
if crc == 'YES':
temp_line = lines[1].strip()
temperature = float(temp_line.split('=')[1])/1000.0
return temperature
else:
return None
except:
return None
```
以上代码中,我们首先初始化DS18B20传感器,然后通过`read_temperature()`函数读取传感器数据并返回温度值。请注意,这里需要将28-00000xxxxxxx替换为你的传感器ID。
接下来,我们可以将采集到的数据以msv格式输出。代码如下:
```python
import time
# 采集并输出数据
with open('data.msv', 'w') as f:
while True:
temperature = read_temperature()
if temperature is not None:
timestamp = time.time()
f.write('{:.3f}, {:.1f}\n'.format(timestamp, temperature))
f.flush()
time.sleep(1)
```
以上代码中,我们不断采集数据并将数据以msv格式写入到`data.msv`文件中。每行数据由时间戳和温度值组成,用逗号分隔。文件写入后,需要调用`flush()`方法将数据写入磁盘。
需要注意的是,`time.time()`返回的是从1970年1月1日开始计算的秒数,需要将其转换为毫秒数以符合msv格式的要求。
希望以上代码可以帮助你采集传感器数据并以msv格式输出。
阅读全文
相关推荐
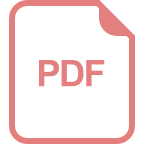
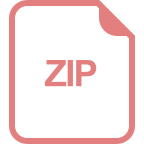


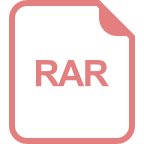
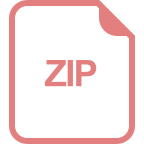
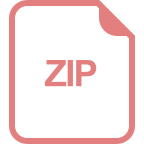
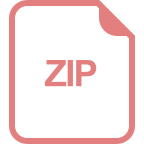
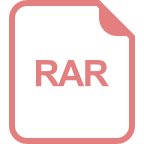
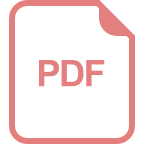

