写给我一个可以通过头插法创建单链表且有合并链表的函数的C语言程序
时间: 2024-05-25 11:17:23 浏览: 96
#include <stdio.h>
#include <stdlib.h>
// 定义链表结构体
struct node {
int data;
struct node *next;
};
// 创建单链表
struct node *createList() {
struct node *head = NULL;
struct node *tail = NULL;
int num;
printf("请输入链表节点值,以-1结束:\n");
scanf("%d", &num);
while (num != -1) {
struct node *new_node = (struct node *) malloc(sizeof(struct node));
new_node->data = num;
new_node->next = NULL;
if (head == NULL) {
head = new_node;
tail = new_node;
} else {
tail->next = new_node;
tail = new_node;
}
scanf("%d", &num);
}
return head;
}
// 合并两个链表
struct node *mergeList(struct node *list1, struct node *list2) {
struct node *head = NULL;
struct node *tail = NULL;
while (list1 != NULL && list2 != NULL) {
if (list1->data <= list2->data) {
if (head == NULL) {
head = list1;
tail = list1;
} else {
tail->next = list1;
tail = list1;
}
list1 = list1->next;
} else {
if (head == NULL) {
head = list2;
tail = list2;
} else {
tail->next = list2;
tail = list2;
}
list2 = list2->next;
}
}
if (list1 != NULL) {
tail->next = list1;
} else {
tail->next = list2;
}
return head;
}
// 打印链表
void printList(struct node *head) {
printf("链表的值为:");
while (head != NULL) {
printf("%d ", head->data);
head = head->next;
}
printf("\n");
}
int main() {
printf("创建第一个链表:\n");
struct node *list1 = createList();
printList(list1);
printf("创建第二个链表:\n");
struct node *list2 = createList();
printList(list2);
printf("合并两个链表:\n");
struct node *merged_list = mergeList(list1, list2);
printList(merged_list);
return 0;
}
阅读全文
相关推荐
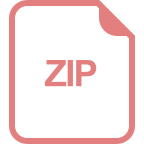
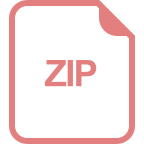
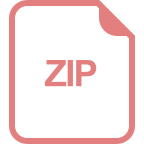
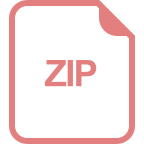
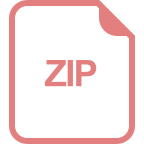
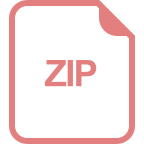
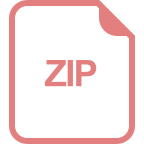
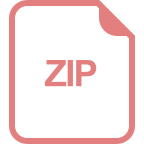
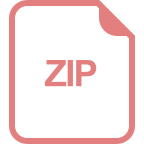
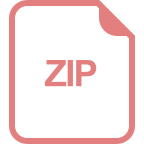
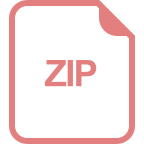
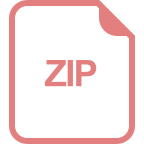
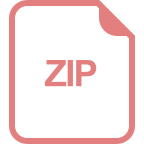
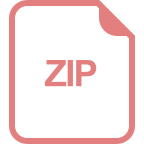
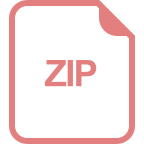