使用Linux虚拟文件系统中的open、read、close接口对某一文件进行读取操作
时间: 2023-12-13 18:24:31 浏览: 18
在Linux虚拟文件系统中,可以使用open、read和close接口对某一文件进行读取操作。下面是一个简单的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <fcntl.h>
#include <unistd.h>
int main()
{
int fd;
char buffer[1024];
ssize_t bytes_read;
fd = open("example.txt", O_RDONLY);
if (fd == -1) {
perror("open");
exit(1);
}
bytes_read = read(fd, buffer, sizeof(buffer));
if (bytes_read == -1) {
perror("read");
exit(1);
}
printf("Read %ld bytes: %.*s\n", bytes_read, (int)bytes_read, buffer);
if (close(fd) == -1) {
perror("close");
exit(1);
}
return 0;
}
```
在示例代码中,首先通过open函数打开一个名为example.txt的文件,并指定只读模式(O_RDONLY)。如果打开失败,则会输出错误信息并退出程序。接着使用read函数读取文件内容,将读取到的数据存储在buffer中。如果读取失败,则同样会输出错误信息并退出程序。最后使用close函数关闭文件,释放文件描述符。
相关推荐
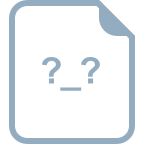
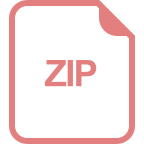
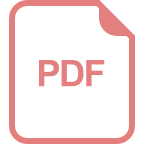














