用C语言写一个算法,计算无头结点的单链表的结点个数
时间: 2024-05-08 10:20:32 浏览: 15
以下是用C语言实现计算无头结点的单链表的结点个数的算法:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义单链表结点结构体
struct Node {
int data;
struct Node* next;
};
// 计算单链表结点个数的函数
int countNodes(struct Node* head) {
int count = 0;
struct Node* currentNode = head;
while (currentNode != NULL) {
count++;
currentNode = currentNode->next;
}
return count;
}
int main() {
// 创建一个单链表
struct Node* head = (struct Node*)malloc(sizeof(struct Node));
head->data = 1;
head->next = NULL;
struct Node* node1 = (struct Node*)malloc(sizeof(struct Node));
node1->data = 2;
node1->next = NULL;
head->next = node1;
struct Node* node2 = (struct Node*)malloc(sizeof(struct Node));
node2->data = 3;
node2->next = NULL;
node1->next = node2;
// 计算单链表结点个数
int count = countNodes(head);
printf("The number of nodes in the linked list is %d.\n", count);
// 释放单链表内存
free(node2);
free(node1);
free(head);
return 0;
}
```
在这个算法中,我们先定义了一个单链表结点结构体 `Node` ,包含一个整数类型的数据成员 `data` 和一个指向下一个结点的指针成员 `next` 。
然后,我们定义了一个计算无头结点的单链表的结点个数的函数 `countNodes` ,该函数接受一个指向单链表头结点的指针作为参数,逐个遍历单链表中的结点,并且使用一个计数器变量 `count` 记录结点个数。
在 `main` 函数中,我们首先创建了一个包含三个结点的单链表,并且调用 `countNodes` 函数计算结点个数,最后释放单链表内存。
输出结果为:
```
The number of nodes in the linked list is 3.
```
相关推荐
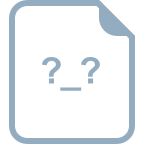
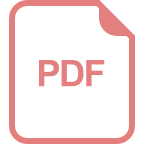














