用c语言设计一个算法,求一个不带头结点的单链表中的结点个数
时间: 2024-09-25 08:03:06 浏览: 37
在C语言中,你可以通过遍历链表的方式来计算其节点个数。这里提供一种简单直接的方法:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
typedef struct Node {
int data;
struct Node* next;
} Node;
// 函数声明:返回链表的节点个数
int countNodes(Node* head) {
if (head == NULL) { // 如果链表为空,返回0
return 0;
} else {
int count = 1; // 初始化计数器
Node* current = head;
while (current->next != NULL) { // 遍历链表直到结束
count++;
current = current->next;
}
return count; // 返回节点总数
}
}
int main() {
// 创建链表示例(此处省略)
Node* head = ...;
int nodeCount = countNodes(head);
printf("链表中的节点个数为:%d\n", nodeCount);
return 0;
}
```
在这个程序中,`countNodes`函数接受链表头节点作为参数,然后通过递增计数器并在每个节点之间移动指针,直到遇到链表的末尾(即`next`为NULL)。最后返回计数器的值就是链表的节点个数。
阅读全文
相关推荐
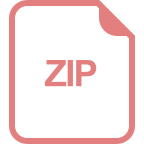
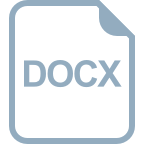
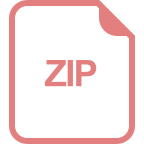















