数据结构线性表C语言,设计一个算法,求一个不带头结点的单链表中的结点个数
时间: 2024-09-28 22:15:46 浏览: 17
在C语言中,你可以通过遍历链表的方式来计算其结点个数,因为每个结点都有一个指向下一个结点的指针,除非链表为空。下面是一个简单的算法实现:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
typedef struct Node {
int data; // 节点存储的数据
struct Node* next; // 指向下一个节点的指针
} Node;
// 函数声明,用于计算链表长度
int countNodes(Node* head);
// 主函数示例
int main() {
// 创建链表并插入数据 (这里仅作演示,实际应用需根据实际情况填充)
Node* head = malloc(sizeof(Node));
Node* second = malloc(sizeof(Node));
Node* third = malloc(sizeof(Node));
head->data = 1;
head->next = second;
second->data = 2;
second->next = third;
third->data = 3;
third->next = NULL;
// 计算链表长度
int length = countNodes(head);
printf("链表中有 %d 个结点。\n", length);
return 0;
}
// 遍历链表并计数
int countNodes(Node* head) {
if (head == NULL) { // 如果链表为空,返回0
return 0;
} else {
int count = 1; // 初始化计数为1
Node* temp = head;
while (temp != NULL) { // 当temp不为空,继续遍历
count++;
temp = temp->next;
}
return count;
}
}
```
在这个算法中,`countNodes` 函数接受链表头节点作为输入,然后通过迭代访问每个节点,直到遇到`NULL`(表示链表结束)。每遇到一个非空节点,计数就加一。
相关推荐
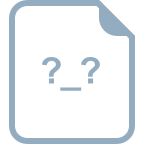
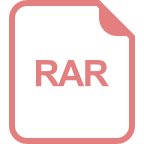
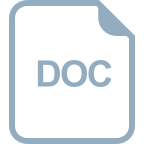














