用C语言设计一个通信录,用顺序存储结构进行存储,设计出生成线性表、在第1个结点位置插入结点、删除第 I 个结点、打印表中结点的算法。
时间: 2024-09-17 18:08:00 浏览: 62
在C语言中,我们可以创建一个简单的通信录数据结构,使用顺序存储(数组或动态内存分配)来存储联系人信息。下面是一个基础的示例,包含生成线性表、插入、删除和打印操作:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义结构体表示联系人
typedef struct {
char name[50];
char phone_number[20];
} Contact;
// 初始化空的通信录
void createContactList(Contact* list, int capacity) {
list = (Contact*)malloc(capacity * sizeof(Contact));
if (!list) {
printf("Memory allocation failed.\n");
return;
}
list[0].name[0] = '\0';
list[0].phone_number[0] = '\0';
}
// 在第一个位置插入新联系人
void insertContactAtFirst(Contact* list, const char* name, const char* phone_number, int capacity) {
if (capacity == 0) {
printf("Contact list is empty.\n");
return;
}
list[0].name = name;
list[0].phone_number = phone_number;
// 如果需要扩容,可以考虑在此处增加处理,这里简单忽略
}
// 删除第I个节点(假设从0开始计数)
void deleteContactAtIndex(Contact* list, int index, int capacity) {
if (index >= 0 && index < capacity - 1) {
memmove(&list[index+1], &list[index], sizeof(Contact)*(capacity-1-index));
free(list[capacity-1]);
list[capacity-1].name[0] = '\0';
list[capacity-1].phone_number[0] = '\0';
} else {
printf("Invalid index.\n");
}
}
// 打印所有联系人信息
void printContactList(const Contact* list, int size) {
for (int i = 0; i < size; i++) {
printf("Name: %s, Phone Number: %s\n", list[i].name, list[i].phone_number);
}
}
int main() {
Contact contactList[10]; // 假设初始容量为10
createContactList(contactList, 10);
// 插入、删除和打印操作...
return 0;
}
```
阅读全文
相关推荐
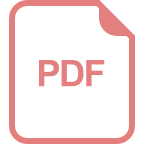
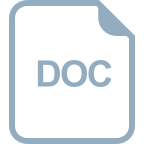
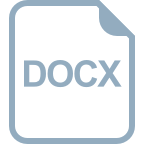















