写出使用十字链表结构实现深度优先遍历的c++代码
时间: 2023-02-11 10:23:16 浏览: 126
```c
void DFS(Node* root) {
Node* p = root;
printf("%d ", p->data);
if (p->firstChild != NULL) {
DFS(p->firstChild);
}
if (p->nextSibling != NULL) {
DFS(p->nextSibling);
}
}
```
其中,Node 结构体中包含三个指针:
- `int data`:节点的数据值
- `Node* firstChild`:指向第一个子节点的指针
- `Node* nextSibling`:指向下一个兄弟节点的指针
代码中,递归函数 DFS() 接受一个 Node 指针作为参数,输出该节点的数据,然后分别访问它的第一个子节点和下一个兄弟节点。
在外部调用时,传入根节点即可:
```c
int main() {
DFS(root);
return 0;
}
```
这个代码是深度优先遍历,那么如果是宽度优先遍历,那就需要使用队列进行实现.
```c
void BFS(Node* root) {
queue<Node*> q;
Node* p = root;
printf("%d ", p->data);
q.push(p);
while (!q.empty()) {
Node* temp = q.front();
q.pop();
p = temp->firstChild;
while (p != NULL) {
printf("%d ", p->data);
q.push(p);
p = p->nextSibling;
}
}
}
```
阅读全文
相关推荐
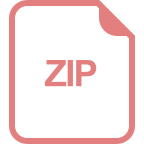
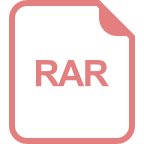
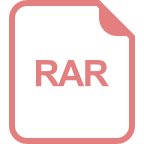
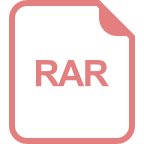
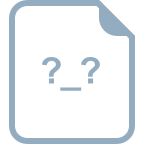
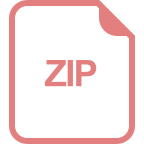
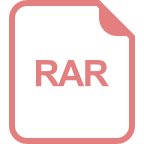
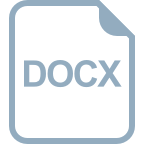
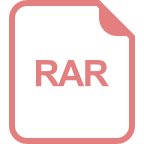
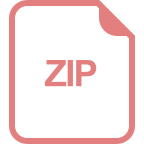
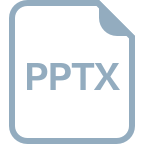
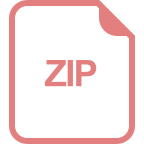
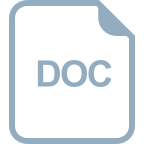
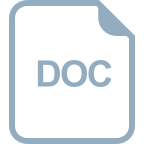
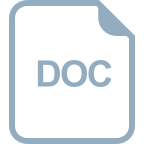
