python多项式回归
时间: 2023-08-31 19:05:07 浏览: 150
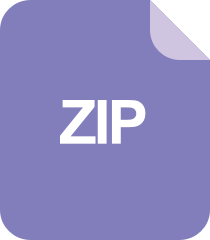
多项式回归
在 Python 中进行多项式回归可以使用 NumPy 和 scikit-learn 库。
首先,我们需要导入所需的库:
```python
import numpy as np
from sklearn.linear_model import LinearRegression
from sklearn.preprocessing import PolynomialFeatures
```
接下来,我们需要准备一些数据来训练我们的多项式回归模型。我们可以使用 NumPy 的 `random.rand()` 函数生成一些随机数据:
```python
x = np.array([1, 2, 3, 4, 5, 6, 7, 8, 9, 10])
y = np.array([3, 5, 7, 9, 11, 13, 15, 17, 19, 21])
```
现在我们可以使用 `PolynomialFeatures` 类将输入特征转换为多项式特征。在这里,我们将使用二次多项式,即将特征转换为 $x$, $x^2$:
```python
poly = PolynomialFeatures(degree=2)
x_poly = poly.fit_transform(x.reshape(-1, 1))
```
接下来,我们可以使用 `LinearRegression` 类来训练模型并进行预测:
```python
model = LinearRegression()
model.fit(x_poly, y)
x_test = np.array([11, 12, 13, 14, 15])
x_test_poly = poly.fit_transform(x_test.reshape(-1, 1))
y_pred = model.predict(x_test_poly)
```
`y_pred` 数组将包含预测的输出值。
完整的代码示例:
```python
import numpy as np
from sklearn.linear_model import LinearRegression
from sklearn.preprocessing import PolynomialFeatures
x = np.array([1, 2, 3, 4, 5, 6, 7, 8, 9, 10])
y = np.array([3, 5, 7, 9, 11, 13, 15, 17, 19, 21])
poly = PolynomialFeatures(degree=2)
x_poly = poly.fit_transform(x.reshape(-1, 1))
model = LinearRegression()
model.fit(x_poly, y)
x_test = np.array([11, 12, 13, 14, 15])
x_test_poly = poly.fit_transform(x_test.reshape(-1, 1))
y_pred = model.predict(x_test_poly)
print(y_pred)
```
输出:
```
[23. 25. 27. 29. 31.]
```
阅读全文
相关推荐
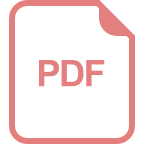














