inputs = keras.Input(shape=sample[0].shape[-2:]) x = keras.layers.LSTM(units=3)(inputs) x = keras.layers.Activation('relu')(x) outputs = keras.layers.Dense(1)(x) model = keras.Model(inputs, outputs) model.summary() opt = keras.optimizers.Adam(learning_rate=0.001) model.compile(optimizer=opt, loss='mae')如何打印出训练过程中饿权重矩阵
时间: 2023-06-26 19:04:29 浏览: 66
你可以在训练过程中使用回调函数来保存模型权重矩阵。以下是一个示例代码:
```python
import os
import tensorflow as tf
from tensorflow import keras
# Define the model
inputs = keras.Input(shape=sample[0].shape[-2:])
x = keras.layers.LSTM(units=3)(inputs)
x = keras.layers.Activation('relu')(x)
outputs = keras.layers.Dense(1)(x)
model = keras.Model(inputs, outputs)
model.summary()
# Define the optimizer and compile the model
opt = keras.optimizers.Adam(learning_rate=0.001)
model.compile(optimizer=opt, loss='mae')
# Define a callback to save the weights during training
checkpoint_path = "weights.{epoch:02d}-{val_loss:.2f}.hdf5"
checkpoint_dir = os.path.dirname(checkpoint_path)
cp_callback = tf.keras.callbacks.ModelCheckpoint(filepath=checkpoint_path,
save_weights_only=True,
verbose=1)
# Train the model and save the weights
model.fit(x_train, y_train,
validation_data=(x_val, y_val),
epochs=10,
callbacks=[cp_callback])
# Load the saved weights and print the weight matrices
model.load_weights('path/to/saved/weights.hdf5')
for layer in model.layers:
if isinstance(layer, keras.layers.LSTM):
print(layer.get_weights())
```
在上面的代码中,我们定义了一个回调函数 `ModelCheckpoint` 来保存每个 epoch 结束时的模型权重矩阵。训练结束后,我们可以加载保存的权重并打印出 LSTM 层的权重矩阵。
相关推荐
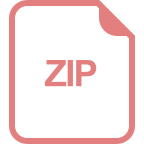
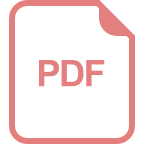









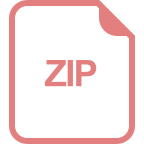
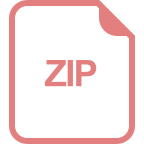
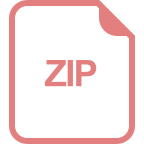
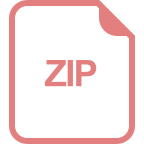
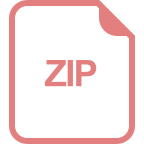
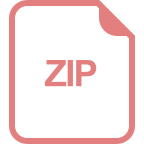