from flask import Flask, request app = Flask(__name__) @app.route('/', methods=['POST']) def generate_b(): a = request.form['a'] # 根据 a 生成对应的 b b = "Generated result for a: {}".format(a) return b if __name__ == '__main__': app.run(host='0.0.0.0', port=3264)
时间: 2024-04-05 13:33:56 浏览: 18
这是一个使用 Flask 框架的 Python 代码,它创建了一个 Web 应用程序,提供了一个 POST 请求的接口 '/generate_b'。当接收到请求时,它会从请求中获取参数 'a' 的值,根据 'a' 的值生成对应的 'b',并将 'b' 作为响应返回给请求方。其中,生成 'b' 的逻辑未给出,需要根据实际需求自行实现。同时,该应用程序会在本地运行,监听地址为 0.0.0.0,端口号为 3264。
相关问题
from flask import Flask, request app = Flask(__name__) @app.route('/example', methods=['POST']) def example(): json_data = request.get_json() # 检查是否成功解析JSON数据 if not json_data: return '无效的JSON数据', 400 # 获取参数值 param1 = json_data.get('param1') param2 = json_data.get('param2') # 进行其他处理... return '成功' if __name__ == '__main__': app.run(),帮我转成fastapi框架
Sure, here is the equivalent code in FastAPI framework:
```python
from fastapi import FastAPI, HTTPException
from pydantic import BaseModel
app = FastAPI()
class ExampleRequest(BaseModel):
param1: str
param2: int
@app.post('/example')
async def example(request: ExampleRequest):
# 获取参数值
param1 = request.param1
param2 = request.param2
# 进行其他处理...
return {'msg': '成功'}
@app.exception_handler(HTTPException)
async def http_exception_handler(request, exc):
return JSONResponse(content={"detail": exc.detail}, status_code=exc.status_code)
if __name__ == '__main__':
import uvicorn
uvicorn.run(app, host='0.0.0.0', port=8000)
```
Note that we are using Pydantic to define the request body schema, and we are returning a JSON response with the "msg" key. Also, we have defined an exception handler to return the error messages in a JSON format.
from flask import Flask, request from Connect_Port import Port import threading from pyngrok import ngrok from flask_cors import CORS p = Port() # app = Flask(__name__) app = Flask(__name__, static_folder='static', static_url_path='/static') request_data = "" CORS(app) @app.route('/data', methods=['POST', 'CURL', 'GET', 'OPTIONS']) def get_data(): global request_data content_type = request.headers.get('Content-Type') request_data = request.data.decode('utf-8') print(request_data) p.send() # if content_type == 'application/json': # json_data = request.json return f"Hello, Received data: {request_data}" if __name__ == '__main__': p_thread = threading.Thread(target=p.run) p_thread.start() public_url = ngrok.connect(9000) print(public_url) app.run(host='0.0.0.0', port=9000) 翻译代码
这段代码是一个使用 Flask 框架构建的简单服务器应用,它可以接收来自客户端的数据,并将数据发送到另一个模块 `Port` 中进行处理。
代码中使用了 `pyngrok` 模块来创建一个内网穿透,以便从外部访问该服务器应用。`Port` 类是一个自定义的模块,用于处理数据的发送。
以下是翻译后的代码:
```python
from flask import Flask, request
from Connect_Port import Port
import threading
from pyngrok import ngrok
from flask_cors import CORS
p = Port()
app = Flask(__name__, static_folder='static', static_url_path='/static')
request_data = ""
CORS(app)
@app.route('/data', methods=['POST', 'CURL', 'GET', 'OPTIONS'])
def get_data():
global request_data
content_type = request.headers.get('Content-Type')
request_data = request.data.decode('utf-8')
print(request_data)
p.send()
return f"Hello, Received data: {request_data}"
if __name__ == '__main__':
p_thread = threading.Thread(target=p.run)
p_thread.start()
public_url = ngrok.connect(9000)
print(public_url)
app.run(host='0.0.0.0', port=9000)
```
这段代码创建了一个 Flask 应用,并定义了一个 `/data` 的路由,用于接收客户端发送的数据。当接收到数据时,会将其发送到 `Port` 模块进行处理。
在 `if __name__ == '__main__':` 代码块中,创建了一个名为 `p_thread` 的线程,用于运行 `Port` 模块的 `run` 方法。然后使用 `ngrok` 创建了一个公开的 URL,以便从外部访问该服务器应用。最后,通过调用 `app.run` 启动了 Flask 应用,监听在本地的 9000 端口上。
请注意,这段代码中引用了一个名为 `Connect_Port` 的模块,你可能需要自行导入或编写该模块。另外,使用 `pyngrok` 进行内网穿透需要提前安装该模块,并在系统中配置 ngrok 的路径。
相关推荐
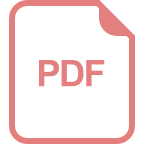
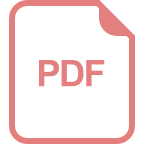
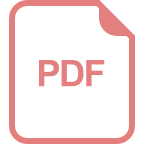













