设计一个动作冒险类游戏 1.游戏里有多种职业角色供玩家使用,比如战士号、法师、猎人等。 2.游戏里有多种武器,比如斧头、弓、法杖 3.每个角色一次只能使用一种武器,但可以在游戏中换武器。 使用c#程序设计语言,实现你的程序架构
时间: 2024-01-24 15:18:49 浏览: 48
游戏架构设计:
1. 游戏角色类设计
```csharp
class Character
{
public string Name { get; set; } // 角色名字
public int Level { get; set; } // 角色等级
public int Health { get; set; } // 角色生命值
public int Mana { get; set; } // 角色魔法值
public int Strength { get; set; } // 角色力量值
public int Intelligence { get; set; } // 角色智力值
public int Agility { get; set; } // 角色敏捷值
public Weapon Weapon { get; set; } // 角色武器
}
```
2. 游戏武器类设计
```csharp
abstract class Weapon
{
public string Name { get; set; } // 武器名称
public int Damage { get; set; } // 武器伤害值
public abstract void Attack(); // 攻击方法
}
class Axe : Weapon
{
public override void Attack()
{
Console.WriteLine("砍!造成 " + Damage + " 点伤害!");
}
}
class Bow : Weapon
{
public override void Attack()
{
Console.WriteLine("射!造成 " + Damage + " 点伤害!");
}
}
class Wand : Weapon
{
public override void Attack()
{
Console.WriteLine("咒!造成 " + Damage + " 点伤害!");
}
}
```
3. 游戏职业类设计
```csharp
abstract class Job
{
public string Name { get; set; } // 职业名称
public abstract Weapon GetWeapon(); // 获取职业对应武器
}
class Warrior : Job
{
public Warrior()
{
Name = "战士";
}
public override Weapon GetWeapon()
{
return new Axe { Name = "斧头", Damage = 10 };
}
}
class Hunter : Job
{
public Hunter()
{
Name = "猎人";
}
public override Weapon GetWeapon()
{
return new Bow { Name = "弓", Damage = 8 };
}
}
class Mage : Job
{
public Mage()
{
Name = "法师";
}
public override Weapon GetWeapon()
{
return new Wand { Name = "法杖", Damage = 12 };
}
}
```
4. 游戏主程序设计
```csharp
class Program
{
static void Main(string[] args)
{
Character character = new Character
{
Name = "小明",
Level = 1,
Health = 100,
Mana = 100,
Strength = 10,
Intelligence = 10,
Agility = 10
};
// 选择职业
Console.WriteLine("请选择职业:");
Console.WriteLine("1. 战士");
Console.WriteLine("2. 猎人");
Console.WriteLine("3. 法师");
int jobIndex = int.Parse(Console.ReadLine()) - 1;
Job[] jobs = { new Warrior(), new Hunter(), new Mage() };
Job job = jobs[jobIndex];
// 装备武器
character.Weapon = job.GetWeapon();
Console.WriteLine("你选择了 " + job.Name + ",装备了 " + character.Weapon.Name);
// 进入游戏
Console.WriteLine("游戏开始!");
while (true)
{
Console.WriteLine("请选择操作:");
Console.WriteLine("1. 攻击");
Console.WriteLine("2. 换武器");
int input = int.Parse(Console.ReadLine());
if (input == 1)
{
character.Weapon.Attack();
}
else if (input == 2)
{
Console.WriteLine("请选择新武器:");
Console.WriteLine("1. 斧头");
Console.WriteLine("2. 弓");
Console.WriteLine("3. 法杖");
int weaponIndex = int.Parse(Console.ReadLine()) - 1;
Weapon[] weapons = { new Axe { Name = "斧头", Damage = 10 }, new Bow { Name = "弓", Damage = 8 }, new Wand { Name = "法杖", Damage = 12 } };
character.Weapon = weapons[weaponIndex];
Console.WriteLine("你换了 " + character.Weapon.Name);
}
}
}
}
```
相关推荐
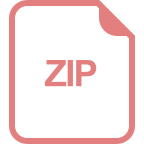
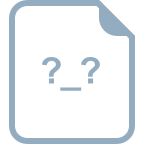















