使用rsa私钥解密文本c语言
时间: 2023-06-30 17:01:53 浏览: 113
### 回答1:
在C语言中使用RSA私钥解密文本的步骤如下:
1. 首先,需要获取RSA私钥,私钥通常以文件的形式存储。使用C语言的文件读取函数,如fopen和fread,读取私钥文件的内容,并将其存储在一个变量中。
2. 将密文文本也读取到一个变量中。
3. 使用C语言的RSA库,加载私钥。可以使用openssl库或者其他类似的库。根据加载私钥的函数接口,将存储私钥的变量作为参数传递给该函数。加载成功后,私钥将被存储在内存中的一个结构体中,可以进行后续的解密操作。
4. 使用加载的私钥,调用解密函数对密文进行解密。解密函数的接口可能会要求传入待解密的密文以及一些其他选项。根据具体的库和函数接口,将存储密文的变量以及其他选项作为参数传递给解密函数。解密函数将返回明文信息。
5. 将解密后的明文信息进行处理,比如打印到屏幕上或存储到一个文件中。
需要注意的是,RSA私钥解密的过程中可能会涉及到一些异常处理的操作,比如加解密错误、内存不足等。因此,在实际的C语言代码中,需要进行适当的错误处理和异常判断。
以上是使用C语言进行RSA私钥解密文本的基本步骤,具体的实现和细节可能会根据所采用的RSA库和函数接口而有所差异。
### 回答2:
使用RSA私钥解密文本在C语言中可以通过以下步骤完成。
首先,我们需要将私钥及待解密的密文导入到C程序中。私钥通常以PEM格式存储在一个文件中,我们可以使用OpenSSL库中的函数来读取私钥文件并将其存储在内存中。
接着,我们需要使用OpenSSL库中的函数将PEM格式的私钥转换为RSA结构体。然后,我们可以使用RSA结构体中的私钥成员变量进行解密操作。
在读取待解密的密文之后,我们可以使用RSA私钥对其进行解密操作。使用OpenSSL库中提供的函数,我们可以将密文中的每个密文块(通常为128字节)传递给解密函数,并将解密后的明文保存在一个缓冲区中。
解密过程完成后,我们就可以使用得到的明文数据进行进一步的处理。如果需要保存解密后的明文到文件中,我们可以使用文件操作相关的函数来实现。
需要注意的是,在使用OpenSSL库的过程中,我们需要包含相关的头文件,并链接相应的库文件。
总结起来,使用RSA私钥解密文本在C语言中的主要步骤包括:导入私钥文件、转换私钥格式、读取待解密的密文、使用私钥进行解密、处理解密后的明文。当然,为了更好地理解整个过程以及具体实现方式,建议查阅相关的C语言和OpenSSL库的文档及示例代码。
### 回答3:
在C语言中,要使用RSA私钥解密文本,可以使用OpenSSL库来实现。下面是一个简单的步骤:
1. 引入OpenSSL库:
```c
#include <openssl/rsa.h>
#include <openssl/pem.h>
```
2. 读取私钥文件:
```c
FILE* file = fopen("private_key.pem", "rb");
RSA* rsa = RSA_new();
rsa = PEM_read_RSAPrivateKey(file, &rsa, NULL, NULL);
fclose(file);
```
3. 读取密文并解密:
```c
unsigned char* ciphertext; // 存储密文的一个字符数组
int ciphertext_len; // 密文长度
unsigned char* plaintext; // 存储解密后的明文的一个字符数组
// TODO: 从文件或其他来源读取密文,并获取其长度
int plaintext_len = RSA_private_decrypt(ciphertext_len, ciphertext, plaintext, rsa, RSA_PKCS1_PADDING);
```
4. 处理解密结果:
```c
if (plaintext_len == -1) {
// 解密失败
ERR_print_errors_fp(stderr);
// 可以添加处理错误的逻辑
} else {
// 解密成功
printf("解密后的明文:\n%s\n", plaintext);
}
// 内存释放
RSA_free(rsa);
free(ciphertext);
free(plaintext);
```
上述代码仅为示例,实际使用中可能需要进行适当的修改和改进。同时,还需要确保正确引入OpenSSL库,并正确配置编译环境。
相关推荐
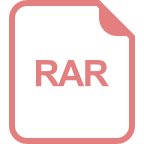
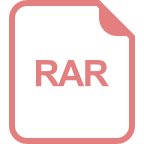














