c语言实现RSA加密解密算法的完整代码
时间: 2024-11-15 09:14:16 浏览: 13
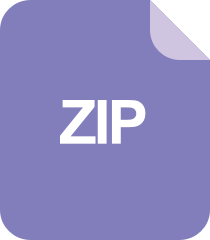
C语言中的 RSA加密和解密算法-_RSA加解密算法的演示,C语言实现

在C语言中,实现RSA加密解密算法通常涉及到大数运算库,比如`gmp.h`或者`openssl`库。下面是一个简单的RSA加密解密函数的概述,使用了模指数运算:
```c
#include <stdio.h>
#include <stdlib.h>
#include "gmp.h"
// RSA公钥和私钥生成
void generate_keys(gmp_int *n, gmp_int *e, gmp_int *d) {
// ... (生成大素数p, q, 计算n = p*q, phi(n), 然后选择合适的e和计算d)
}
// RSA加密
void rsa_encrypt(unsigned char *plaintext, size_t plaintext_len, gmp_int *n, gmp_int *e, unsigned char *ciphertext) {
mpz_t ciphertext_mpz;
mpz_init_set_ui(ciphertext_mpz, 0); // 初始化大整数
for (size_t i = 0; i < plaintext_len; ++i) {
mpz_powm(ciphertext_mpz, ciphertext_mpz, mpz_get_ui(plaintext + i), n, e);
}
// 将大整数转换回字节并复制到结果数组
ciphertext = mpz_get_str(ciphertext, 256, ciphertext_mpz);
mpz_clear(ciphertext_mpz);
}
// RSA解密
void rsa_decrypt(unsigned char *ciphertext, size_t ciphertext_len, gmp_int *n, gmp_int *d, unsigned char *plaintext) {
mpz_t plaintext_mpz;
mpz_init_set_ui(plaintext_mpz, 0);
mpz_powm(plaintext_mpz, ciphertext, d, n, NULL);
// 解密后的字节数组处理
plaintext = mpz_get_str(plaintext, 256, plaintext_mpz);
mpz_clear(plaintext_mpz);
}
int main() {
// 实例化大整数变量
gmp_int *n, *e, *d;
// 生成公钥和私钥
generate_keys(&n, &e, &d);
// 加密示例
unsigned char plaintext[] = {0x48, 0x65, 0x6c, 0x6c, 0x6f};
unsigned char ciphertext[1024];
rsa_encrypt(plaintext, sizeof(plaintext), n, e, ciphertext);
// 解密示例
unsigned char decrypted_text[1024];
rsa_decrypt(ciphertext, strlen((char*)ciphertext), n, d, decrypted_text);
// 输出解密后的文本
printf("Decrypted text: %s\n", decrypted_text);
// 清理资源
mpz_clear(d);
mpz_clear(e);
mpz_clear(n);
return 0;
}
```
注意:这只是一个简化版本,实际应用中需要考虑错误检查、边界条件、安全性和性能优化。此外,这个例子假设您已经安装了`gmp`库并且熟悉其API。
阅读全文
相关推荐
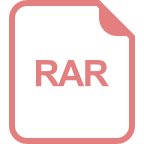


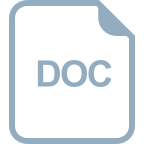
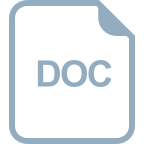
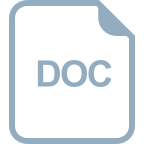
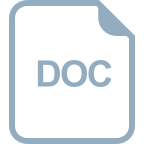
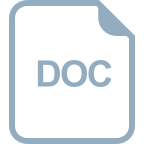





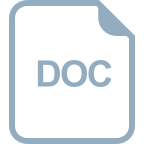
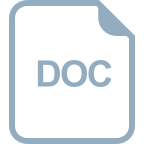
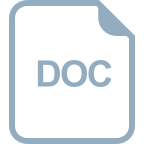

