vue模拟微信聊天头像怎么写
时间: 2024-02-05 12:03:13 浏览: 109
要模拟微信聊天头像,可以使用 Vue 组件的方式来实现。具体步骤如下:
1. 在 HTML 中引入一个 img 标签,设置它的样式为圆形,并且设置它的宽高为相同值,比如 50px:
```html
<template>
<img class="avatar" :src="avatarUrl">
</template>
<style>
.avatar {
width: 50px;
height: 50px;
border-radius: 50%;
}
</style>
```
2. 在 Vue 组件中,定义一个名为 avatarUrl 的属性,用于存储头像的 URL 地址:
```javascript
<script>
export default {
data() {
return {
avatarUrl: 'https://example.com/avatar.jpg'
}
}
}
</script>
```
3. 如果需要通过 props 传递头像地址,则可以在组件中使用 props 接收该值:
```javascript
<script>
export default {
props: {
avatarUrl: {
type: String,
required: true
}
}
}
</script>
```
这样就可以通过传递不同的头像地址来显示不同的头像了。
相关问题
vue模拟微信聊天界面,输入任何文字统一回复‘你好“ 的完整代码
以下是一个简单的 Vue.js 实现模拟微信聊天界面,输入任何文字统一回复‘你好’的完整代码:
```
<template>
<div class="chat-room">
<div class="chat-container">
<div class="chat-msg" v-for="(msg, index) in messages" :key="index">
<div class="chat-avatar">
<img :src="msg.avatar" alt="avatar" />
</div>
<div class="chat-content">
<div class="chat-info">
<span class="chat-name">{{ msg.name }}</span>
<span class="chat-time">{{ msg.time }}</span>
</div>
<div class="chat-text">{{ msg.text }}</div>
</div>
</div>
</div>
<div class="chat-input">
<input type="text" v-model="inputText" @keyup.enter="sendMessage" />
<button @click="sendMessage">发送</button>
</div>
</div>
</template>
<script>
export default {
name: "ChatRoom",
data() {
return {
inputText: "",
messages: [
{
avatar: "https://i.pravatar.cc/50?img=1",
name: "小明",
time: "2021-10-01 12:00",
text: "你在干嘛?",
},
{
avatar: "https://i.pravatar.cc/50?img=2",
name: "小红",
time: "2021-10-01 12:05",
text: "我在看电影,你呢?",
},
{
avatar: "https://i.pravatar.cc/50?img=3",
name: "小明",
time: "2021-10-01 12:10",
text: "我在写代码",
},
],
};
},
methods: {
sendMessage() {
if (this.inputText.trim() === "") return;
const time = new Date().toLocaleString();
const newMessage = {
avatar: "https://i.pravatar.cc/50?img=4",
name: "我",
time,
text: this.inputText,
};
this.messages.push(newMessage);
this.inputText = "";
setTimeout(() => {
const reply = {
avatar: "https://i.pravatar.cc/50?img=5",
name: "小机器人",
time: new Date().toLocaleString(),
text: "你好",
};
this.messages.push(reply);
}, 1000);
},
},
};
</script>
<style>
.chat-room {
height: 100vh;
display: flex;
flex-direction: column;
}
.chat-container {
height: calc(100% - 50px);
overflow-y: scroll;
padding: 10px;
}
.chat-msg {
display: flex;
margin-bottom: 10px;
}
.chat-avatar {
margin-right: 10px;
}
.chat-avatar img {
width: 50px;
height: 50px;
border-radius: 50%;
}
.chat-info {
display: flex;
justify-content: space-between;
margin-bottom: 5px;
}
.chat-name {
font-weight: bold;
}
.chat-time {
color: #999;
}
.chat-text {
word-wrap: break-word;
}
.chat-input {
display: flex;
justify-content: space-between;
align-items: center;
height: 50px;
background-color: #fafafa;
padding: 10px;
}
.chat-input input {
flex: 1;
height: 30px;
border: none;
border-radius: 5px;
padding: 5px;
}
.chat-input button {
height: 30px;
margin-left: 10px;
}
</style>
```
这段代码实现了一个简单的聊天室界面,包括消息列表、发送消息输入框和发送按钮。其中,消息列表使用 `v-for` 指令遍历 `messages` 数组,每个消息包括头像、昵称、时间和文本内容。发送消息时,通过 `v-model` 指令绑定输入框的值,通过 `@keyup.enter` 监听回车键事件或者点击发送按钮时调用 `sendMessage` 方法,将新消息添加到 `messages` 数组中,并且通过 `setTimeout` 函数模拟了一个简单的自动回复,即在 1 秒后添加一个 “你好” 的消息到 `messages` 数组中。
vue中模拟微信聊天界面的所有代码
以下是一个简单的 Vue 组件,用于模拟微信聊天界面:
```html
<template>
<div class="chat">
<div v-for="(message, index) in messages" :key="index" class="message" :class="{ 'from-me': message.from === 'me', 'from-other': message.from === 'other' }">
<div class="avatar" v-if="message.showAvatar">{{ message.from }}</div>
<div class="bubble">{{ message.content }}</div>
</div>
<div class="input-area">
<textarea v-model="inputText" @keyup.enter="sendMessage" placeholder="Type a message"></textarea>
<button @click="sendMessage">Send</button>
</div>
</div>
</template>
<script>
export default {
data() {
return {
messages: [
{ from: 'me', content: 'Hello!', showAvatar: true },
{ from: 'other', content: 'Hi there!', showAvatar: true },
],
inputText: '',
};
},
methods: {
sendMessage() {
if (!this.inputText) {
return;
}
this.messages.push({ from: 'me', content: this.inputText, showAvatar: false });
this.inputText = '';
},
},
};
</script>
<style>
.chat {
display: flex;
flex-direction: column;
height: 100%;
}
.message {
display: flex;
align-items: flex-end;
margin: 10px;
}
.from-me .avatar {
display: none;
}
.from-other .avatar {
width: 40px;
height: 40px;
margin-right: 10px;
border-radius: 50%;
background-color: #ccc;
display: flex;
justify-content: center;
align-items: center;
}
.bubble {
max-width: 70%;
border-radius: 20px;
padding: 10px;
background-color: #eee;
}
.input-area {
display: flex;
align-items: center;
padding: 10px;
}
textarea {
flex: 1;
height: 30px;
resize: none;
margin-right: 10px;
}
button {
padding: 10px;
background-color: #4caf50;
color: white;
border: none;
border-radius: 5px;
cursor: pointer;
}
</style>
```
这个组件包含一个消息列表和一个输入框,通过 `v-for` 指令来渲染消息列表。每个消息都有一个 `from` 属性,表示是谁发送的,以及一个 `content` 属性,表示消息内容。输入框通过 `v-model` 指令和 `inputText` 数据属性进行双向绑定。
在发送消息时,我们通过 `sendMessage` 方法将消息添加到 `messages` 数组中,并将输入框清空。同时,我们可以根据消息发送者的不同,来决定是否显示头像。
阅读全文
相关推荐



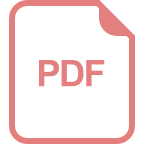
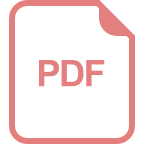
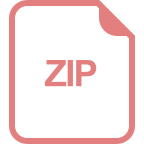
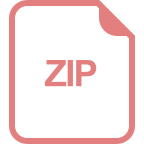
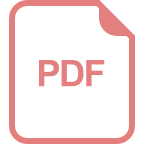
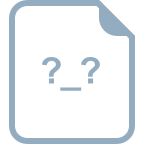
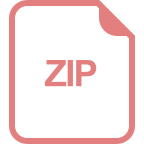
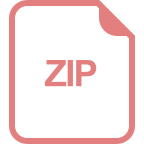
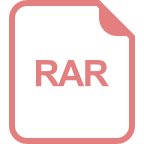
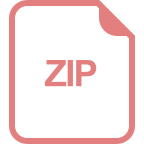
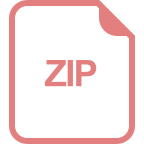
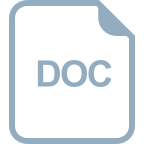