用vue3模拟微信聊天功能显示头像及消息置底后自动上滑的代码
时间: 2024-03-31 07:33:27 浏览: 153
好的,以下是一个用 Vue3 实现微信聊天功能的示例代码,包括显示头像、消息置底和自动上滑。
```html
<template>
<div class="chat">
<div class="chat-content" ref="chatContent">
<div class="message" v-for="message in messages" :key="message.id">
<div class="avatar">
<img :src="message.avatar" alt="avatar">
</div>
<div class="text">{{ message.text }}</div>
</div>
</div>
<div class="input">
<input type="text" v-model="newMessage" @keyup.enter="sendMessage">
<button @click="sendMessage">发送</button>
</div>
</div>
</template>
<script>
import { ref, reactive, watchEffect } from 'vue'
export default {
setup() {
const messages = reactive([
{
id: 1,
avatar: 'https://via.placeholder.com/50',
text: '你好,这是第一条消息'
},
{
id: 2,
avatar: 'https://via.placeholder.com/50',
text: '这是第二条消息'
},
{
id: 3,
avatar: 'https://via.placeholder.com/50',
text: '这是第三条消息'
}
])
const newMessage = ref('')
const sendMessage = () => {
if (!newMessage.value) return
const id = messages.length + 1
const avatar = 'https://via.placeholder.com/50'
const text = newMessage.value
messages.push({ id, avatar, text })
newMessage.value = ''
}
// 消息置底
const chatContent = ref(null)
watchEffect(() => {
chatContent.value.scrollTop = chatContent.value.scrollHeight
})
// 自动上滑
const lastScrollTop = ref(0)
const handleScroll = () => {
const { scrollTop, offsetHeight, scrollHeight } = chatContent.value
if (scrollTop < lastScrollTop.value && scrollTop + offsetHeight < scrollHeight) {
chatContent.value.scrollTop = chatContent.value.scrollHeight
}
lastScrollTop.value = scrollTop
}
return { messages, newMessage, sendMessage, chatContent, handleScroll }
},
mounted() {
this.$refs.chatContent.addEventListener('scroll', this.handleScroll)
},
beforeUnmount() {
this.$refs.chatContent.removeEventListener('scroll', this.handleScroll)
}
}
</script>
<style>
.chat {
height: 500px;
border: 1px solid #ccc;
display: flex;
flex-direction: column;
}
.chat-content {
flex: 1;
overflow-y: auto;
scroll-behavior: smooth;
}
.message {
display: flex;
margin-bottom: 10px;
}
.avatar {
width: 50px;
height: 50px;
margin-right: 10px;
}
.text {
flex: 1;
background-color: #f0f0f0;
padding: 10px;
border-radius: 10px;
}
.input {
display: flex;
justify-content: space-between;
padding: 10px;
}
.input input {
flex: 1;
margin-right: 10px;
}
.input button {
width: 80px;
}
</style>
```
上述代码中,我们使用了 Vue3 的 `reactive` 和 `ref` 方法来创建响应式数据和引用数据,使用 `watchEffect` 来监听 `messages` 的变化,实现消息置底。在 `setup` 函数中,我们还定义了一个 `handleScroll` 方法来监听 `chatContent` 的滚动事件,实现自动上滑。最后,在 `mounted` 和 `beforeUnmount` 生命周期中分别添加和移除 `scroll` 事件监听器,避免内存泄漏。
阅读全文
相关推荐
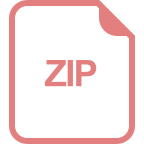




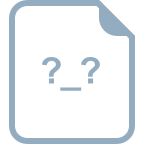
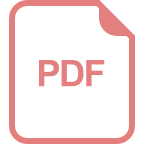

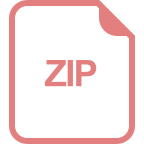
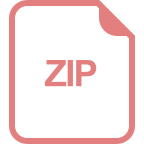
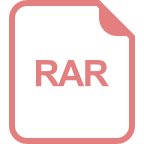
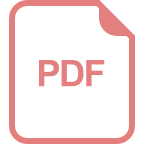
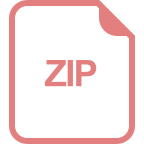
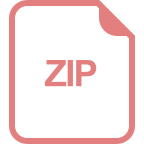
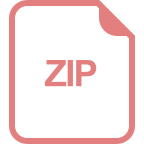
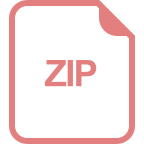
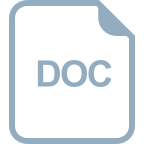