用Java写*编程:写一个函数,接受一个整数,输出这个整数是几位数。
时间: 2024-02-28 08:55:12 浏览: 107
好的,以下是 Java 代码实现:
```java
public static int countDigits(int num) {
/**
* 计算一个整数的位数
*/
int count = 0;
while (num != 0) {
count++;
num /= 10;
}
return count;
}
```
这个函数接受一个整数 `num`,通过不断地将它除以 10,直到它变成 0,来计算它的位数。最后返回位数值。
例如,如果调用 `countDigits(12345)`,函数会返回 5。
相关问题
计算整数位数用什么函数
计算整数的位数通常可以用编程语言提供的内置函数来完成。以下是几种常见编程语言中的方法:
1. **Python**:
```python
len(str(abs(num))) # 使用str()转换为字符串,再用len()获取长度,适用于正负数
```
2. **Java**:
```java
int bitLength = Integer.bitCount(num); // 对于整数,可以使用Integer类的bitCount方法
```
3. **C++**:
```cpp
int countBits(int num) {
return __builtin_popcount(num); // C++17开始支持__builtin_popcount,返回二进制表示中1的个数
}
```
4. **JavaScript**:
```javascript
Math.log10(Math.abs(num)) + 1; // 通过自然对数近似计算位数
```
5. **PHP**:
```php
floor(log10(abs($num))) + 1; // 使用log10函数并向下取整
```
请注意,上述函数都假设输入的是非负整数。对于负数,你需要先取绝对值。另外,不同编程语言可能会有不同的函数或库来实现这一功能。
如何确定一个数字的位数(如:一个整数有几位)?
确定一个数字的位数通常涉及到编程中的数学操作和逻辑判断。这里以几种常见编程语言为例:
1. **Python**:
```python
def get_digits_count(n):
return len(str(abs(n))) # 使用str()函数转换数字为字符串,然后计算长度
num = int(input("请输入一个整数: "))
print(f"{num}的位数为: {get_digits_count(num)}")
```
2. **JavaScript**:
```javascript
function digitsCount(num) {
return num.toString().length;
}
var num = parseInt(prompt("请输入一个整数: "));
console.log(`数字${num}的位数是: ${digitsCount(num)}`);
```
3. **Java**:
```java
public static int countDigits(int n) {
return String.valueOf(Math.abs(n)).length();
}
Scanner scanner = new Scanner(System.in);
System.out.println("请输入一个整数: " + scanner.nextInt());
System.out.println("该数字的位数为: " + countDigits(scanner.nextInt()));
```
4. **C#**:
```csharp
int GetDigitCount(int number)
{
return Math.Abs(number).ToString().Length;
}
Console.WriteLine("请输入一个整数: ");
int input = Convert.ToInt32(Console.ReadLine());
Console.WriteLine($"数字{input}的位数为: {GetDigitCount(input)}");
```
在上述代码中,首先将数字转换为字符串形式,然后通过字符串的`length`属性获取字符(数字)的数量,即位数。
阅读全文
相关推荐
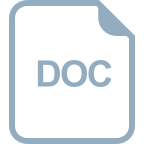
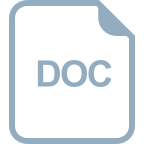
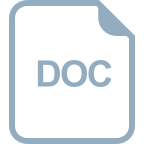
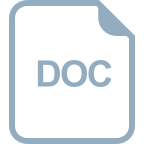

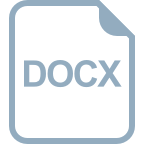
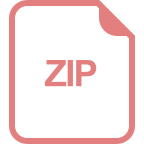
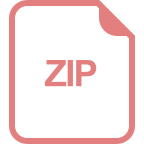
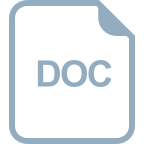
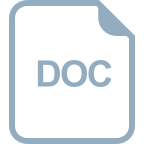
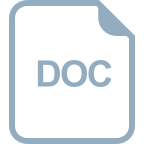
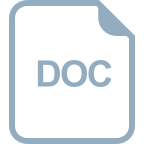
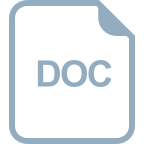
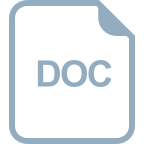