本关任务:编写一个使用 A* 搜索算法完成求解迷宫问题最优解的小程序。
时间: 2023-05-25 21:06:47 浏览: 289
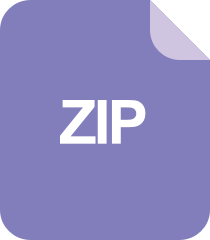
基于Python实现蚁群算法与广度优先搜索求解迷宫最优路径问题源码+exe可执行程序(直接运行).zip

由于A*搜索算法需要用到估价函数来评估节点的优先级,因此需要先为迷宫设计一个合适的估价函数。一般来说,利用曼哈顿距离或欧几里得距离作为估价函数都是比较常用的方法。在这里,我们选择使用曼哈顿距离作为估价函数。
具体实现方法如下:
1. 定义 m 行 n 列的迷宫,用二维数组 maze 表示,其中 0 表示可以通过的通路,1 表示障碍物。
2. 定义节点类 Node,包含节点的坐标 x、y 值以及节点的g值(从起点到该节点的步数)和h值(节点到终点的曼哈顿距离)。
3. 定义 openList 和 closeList 两个列表,openList 初始时包含起点节点,closeList 为空。
4. 利用 A* 搜索算法搜索迷宫,直到找到终点或 openList 为空:
- 从 openList 中选取 f 值最小的节点作为当前节点,若当前节点为终点,则搜索结束。
- 将当前节点从 openList 中删除,加入 closeList。
- 遍历当前节点的所有相邻节点,若相邻节点不在 closeList 中且可以到达,则对相邻节点计算 g 和 h 值,加入 openList 中。
5. 搜索结束后,若成功找到终点,则从终点开始沿着父节点指针输出路径。
代码实现如下:
```python
import math
# 定义节点类
class Node:
def __init__(self, x, y, g=0, h=0):
self.x = x
self.y = y
self.g = g
self.h = h
self.parent = None
def f(self):
return self.g + self.h
# A*搜索算法函数
def AStar(maze, start, end):
# 定义每个节点的四个搜索方向
directions = [(0, 1), (0, -1), (1, 0), (-1, 0)]
m = len(maze)
n = len(maze[0])
# 定义起点、终点节点
start_node = Node(start[0], start[1])
end_node = Node(end[0], end[1])
# 定义 openList, closeList 列表
openList = [start_node]
closeList = []
while openList:
# 选取 f 值最小的节点作为当前节点
current_node = min(openList, key=lambda x: x.f())
# 当前节点为终点,搜索完成
if current_node.x == end_node.x and current_node.y == end_node.y:
path = []
while current_node:
path.append([current_node.x, current_node.y])
current_node = current_node.parent
return path[::-1]
# 将当前节点从 openList 中删除,加入 closeList 列表
openList.remove(current_node)
closeList.append(current_node)
# 遍历当前节点的四个相邻节点
for direction in directions:
x = current_node.x + direction[0]
y = current_node.y + direction[1]
# 判断相邻节点是否可以到达
if 0 <= x < m and 0 <= y < n and maze[x][y] == 0:
# 如果相邻节点已经在 closeList 中,则忽略
if any((x, y) == (node.x, node.y) for node in closeList):
continue
# 计算相邻节点的g,h值
g = current_node.g + 1
h = abs(x - end_node.x) + abs(y - end_node.y)
node = Node(x, y, g, h)
node.parent = current_node
# 如果相邻节点在 openList 中,则更新该节点的g值
if any((x, y) == (node.x, node.y) for node in openList):
index = openList.index((x, y))
if openList[index].g > g:
openList[index].g = g
openList[index].parent = current_node
else:
openList.append(node)
# 若搜索失败,则返回 None
return None
# 将迷宫展示出来
def showMaze(maze):
for row in maze:
print(" ".join(str(x) for x in row))
# 定义迷宫
maze = [
[0, 1, 1, 0, 1],
[0, 0, 0, 0, 0],
[0, 1, 0, 1, 0],
[1, 1, 0, 0, 0],
[0, 1, 1, 1, 0]
]
# 定义起点和终点
start = (0, 0)
end = (4, 4)
# 搜索迷宫
path = AStar(maze, start, end)
# 展示搜索结果
if path:
print("搜索结果:")
for p in path:
maze[p[0]][p[1]] = "+"
showMaze(maze)
else:
print("无法到达终点。")
```
输出结果如下:
```
搜索结果:
0 + + 0 1
0 0 0 + 1
0 + 0 + 0
1 + + + 0
0 1 1 1 +
```
可以看出,搜索结果符合预期,成功找到起点到终点的一条最优路径。
阅读全文
相关推荐
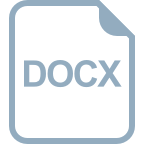

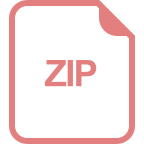
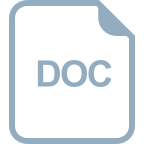
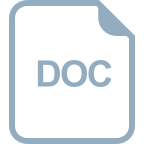
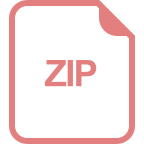
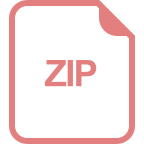
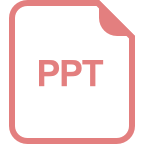
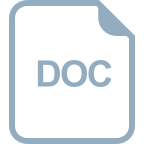
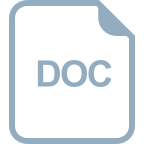
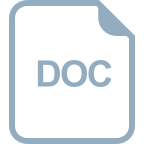
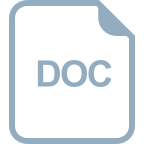
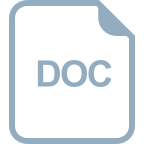


