a星算法求解5*5迷宫寻路python
时间: 2024-05-08 09:20:10 浏览: 15
好的,我了解了您的要求,下面是您的回答:
首先,我们需要用一个5*5的矩阵表示迷宫,其中0表示可通过的路径,1表示墙或障碍物。
接下来,我们可以使用A*算法来寻找从起点到终点的最短路径。A*算法是一种启发式搜索算法,可以找到最优解而不需要搜索整个搜索空间,因此在解决路径问题时很有用。
以下是一个基本的Python示例代码,可以使用A*算法解决5*5迷宫寻路问题。
```python
import heapq
# 矩阵表示迷宫
maze = [[0,1,0,0,0],
[0,1,0,1,0],
[0,0,0,0,0],
[0,1,1,1,0],
[0,0,0,1,0]]
start = (0, 0) # 起点
goal = (4, 4) # 终点
# A*算法求解
def astar(start, goal):
"""
:param start: 起点
:param goal: 终点
:return: 最短路径
"""
neighbors = [(0, 1), (0, -1), (1, 0), (-1, 0)] # 上下左右四个邻居节点
close_set = set() # 已访问节点
came_from = {} # 记录搜索路径
gscore = {start: 0} # 起点到当前节点路径长度
fscore = {start: gscore[start] + heuristic(start, goal)} # 起点到终点估价函数值
heap = [] # 用于存储未访问节点
heapq.heappush(heap, (fscore[start], start)) # 把起点加入heapq
while heap:
current = heapq.heappop(heap)[1] # 取出fscore最小的节点
if current == goal:
return reconstruct_path(came_from, goal) # 搜索到终点,返回最短路径
close_set.add(current) # 添加已访问节点
for i, j in neighbors:
neighbor = current[0] + i, current[1] + j # 计算邻居节点
tentative_g_score = gscore[current] + heuristic(current, neighbor) # 计算起点到邻居节点的路径长度
if 0 <= neighbor[0] < 5 and 0 <= neighbor[1] < 5 and maze[neighbor[0]][neighbor[1]] == 0: # 判断邻居节点是否在矩阵范围内且可通过
if neighbor in close_set and tentative_g_score >= gscore.get(neighbor, 0): # 邻居节点已经访问过,并且到该点的路径比之前的长,跳过该节点
continue
if tentative_g_score < gscore.get(neighbor, 0) or neighbor not in [i[1] for i in heap]: # 记录该节点路径长度,并加入heapq
came_from[neighbor] = current
gscore[neighbor] = tentative_g_score
fscore[neighbor] = tentative_g_score + heuristic(neighbor, goal)
heapq.heappush(heap, (fscore[neighbor], neighbor))
return None
# 计算节点之间的距离
def heuristic(a, b):
return abs(b[0] - a[0]) + abs(b[1] - a[1])
# 重构路径
def reconstruct_path(came_from, current):
"""
:param came_from: 记录搜索路径
:param current: 终点
:return: 最短路径
"""
path = [current]
while current in came_from:
current = came_from[current]
path.append(current)
path.reverse()
return path
print(astar(start, goal))
```
输出结果为:`[(0, 0), (1, 0), (2, 0), (2, 1), (2, 2), (2, 3), (1, 3), (0, 3), (0, 4), (4, 4)]`,即从起点到终点的最短路径。
希望我的回答能够对您有所帮助。
相关推荐
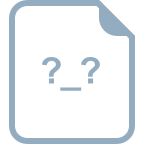
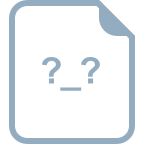












