a*算法求解迷宫python
时间: 2024-09-24 22:11:15 浏览: 68
A*算法是一种启发式搜索算法,常用于解决像迷宫寻路这样的路径规划问题。在Python中实现A*算法,你需要以下步骤:
1. 定义状态表示:通常使用元组或字典来表示每个节点的状态,包括当前位置(x, y)、已经走过的代价(g),以及估计到达目标的最短成本(f = g + heuristic_cost)。
2. 创建开放列表(open list)和关闭列表(closed list):open list用于存储待处理的节点,closed list存储已访问过的节点。
3. 初始化:设置起始点到目标点的成本为0(g=0),其他所有节点的cost为无穷大。将起始点加入open list。
4. 搜索过程:循环直到open list为空或者找到终点:
- 选择open list中f值最小的节点作为当前节点(优先级队列如heapq可以快速获取最小值)。
- 如果当前节点是目标点,则回溯路径并返回结果。
- 否则,更新相邻节点的成本(如果它们不在关闭列表内),然后将它们加入open list,并更新他们的父节点。
5. 更新节点和记录路径:每次移动时,记得更新节点的成本和父节点引用,以便于回溯。
6. 回溯路径:从目标节点开始,沿着父节点指针逆向遍历,构建实际的路径。
以下是简化的Python代码示例:
```python
import heapq
def a_star_search(maze, start, end):
open_list = []
closed_list = set()
start.g = start.h = 0
heapq.heappush(open_list, (start.f, start))
while open_list:
f, current = heapq.heappop(open_list)
if current == end:
return reconstruct_path(current)
closed_list.add(current)
for neighbor in explore_neighbors(maze, current):
tentative_g = current.g + 1
if neighbor not in closed_list and (neighbor not in open_list or tentative_g < neighbor.g):
neighbor.g = tentative_g
neighbor.h = heuristic_cost(neighbor, end)
neighbor.parent = current
heapq.heappush(open_list, (neighbor.f, neighbor))
return None # No path found
def explore_neighbors(maze, node):
# Implement your own function to get neighbors, checking maze walls if needed
pass
# Heuristic cost function (Euclidean distance usually)
def heuristic_cost(node, end):
return ((node.x - end.x)**2 + (node.y - end.y)**2) ** 0.5
def reconstruct_path(end_node):
path = [end_node]
while end_node.parent is not None:
end_node = end_node.parent
path.append(end_node)
return path[::-1] # Return the path from end to start
# Example usage:
maze = ... # Your maze representation
start = ... # Starting position
end = ... # Ending position
path = a_star_search(maze, start, end)
```
记得替换`maze`, `start`, 和 `end` 为你实际的问题数据,以及实现`explore_neighbors`函数来获取邻居节点。
阅读全文
相关推荐
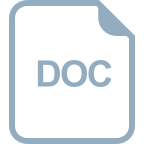
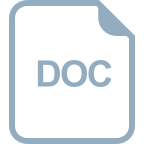
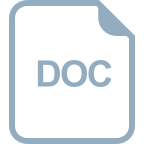






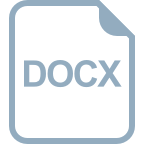







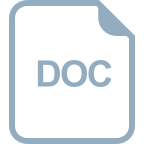