帮我写出一个Java版的走迷宫代码
时间: 2023-05-12 21:04:48 浏览: 107
好的,以下是一个简单的Java版走迷宫代码:
```
import java.util.*;
public class MazeSolver {
private int[][] maze;
private boolean[][] visited;
private int startRow, startCol, endRow, endCol;
public MazeSolver(int[][] maze, int startRow, int startCol, int endRow, int endCol) {
this.maze = maze;
this.startRow = startRow;
this.startCol = startCol;
this.endRow = endRow;
this.endCol = endCol;
visited = new boolean[maze.length][maze[0].length];
}
public boolean solve() {
return solve(startRow, startCol);
}
private boolean solve(int row, int col) {
if (row < 0 || row >= maze.length || col < 0 || col >= maze[0].length || visited[row][col] || maze[row][col] == 1) {
return false;
}
visited[row][col] = true;
if (row == endRow && col == endCol) {
return true;
}
if (solve(row + 1, col) || solve(row - 1, col) || solve(row, col + 1) || solve(row, col - 1)) {
return true;
}
return false;
}
public static void main(String[] args) {
int[][] maze = {
{0, 1, 0, 0},
{0, 1, 0, 1},
{0, 0, 0, 0},
{1, 0, 1, 0}
};
MazeSolver solver = new MazeSolver(maze, 0, 0, 3, 3);
if (solver.solve()) {
System.out.println("Maze solved!");
} else {
System.out.println("Maze cannot be solved.");
}
}
}
```
这个代码使用递归的方式来解决迷宫问题。我们首先定义一个 `MazeSolver` 类,它包含一个迷宫数组 `maze`,起点和终点的行列坐标,以及一个 `visited` 数组来记录哪些位置已经被访问过了。
在 `solve()` 方法中,我们调用 `solve(startRow, startCol)` 来开始递归求解迷宫。在 `solve(row, col)` 方法中,我们首先检查当前位置是否越界、是否已经访问过、是否是障碍物。如果是,直接返回 `false`。否则,我们将当前位置标记为已访问,并检查当前位置是否是终点。如果是,返回 `true`。否则,我们递归地尝试向上、下、左、右四个方向走,如果其中任意一个方向能够到达终点,就返回 `true`。如果四个方向都无法到达终点,返回 `false`。
在 `main()` 方法中,我们定义了一个迷宫数组 `maze`,然后创建一个 `MazeSolver` 对象,并调用 `solve()` 方法来解决迷宫问题。如果能够解决,输出 "Maze solved!",否则输出 "Maze cannot be solved."。
阅读全文
相关推荐


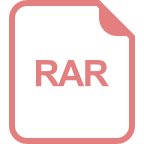


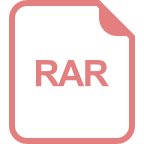









