补全代码:#include <stdio.h> #include <stdlib.h> #include <unistd.h> #include <fcntl.h> #include <time.h> #include <errno.h> #define MAX_BUFFER_SIZE 1024 #define IN_FILES 3 #define TIME_DELAY 60 #define MAX(a, b) ((a > b) ? (a) : (b)) #define IN1 "/data/workspace/myshixun/case3/in1" #define IN2 "/data/workspace/myshixun/case3/in2" int main(void) { int fds[IN_FILES]; char buf[MAX_BUFFER_SIZE]; int i, res, real_read, maxfd; struct timeval tv; fd_set inset, tmp_inset; fds[0] = 0; if ((fds[1] = open(IN1, O_RDONLY | O_NONBLOCK)) < 0) { printf("Open IN1 error.\n"); return 1; } if ((fds[2] = open(IN2, O_RDONLY | O_NONBLOCK)) < 0) { printf("Open IN2 error.\n"); return 1; } maxfd = MAX(fds[1], fds[2]); FD_ZERO(&inset); for (i = 0; i < IN_FILES; i++) FD_SET(fds[i], &inset); tv.tv_sec = TIME_DELAY; tv.tv_usec = 0; while (FD_ISSET(fds[0], &inset) || FD_ISSET(fds[1], &inset) || FD_ISSET(fds[2], &inset)) { tmp_inset = inset; //使用select函数 res = select(); switch (res) { case -1: { printf("Select error\n"); return 1; } break; case 0: { printf("Time out\n"); return 1; } break; default: for (i = 0; i < IN_FILES; i++) { if (FD_ISSET(fds[i], &tmp_inset)) { memset(buf, 0, MAX_BUFFER_SIZE); //读取到buf里 real_read = read(); if (real_read < 0) { if (errno != EAGAIN) return 1; } else if (!real_read) { close(fds[i]); FD_CLR(fds[i], &inset); } else {
时间: 2024-02-10 19:22:27 浏览: 68
//将读取到的内容输出到标准输出
write(1, buf, real_read);
}
}
break;
}
}
}
//关闭文件描述符
for (i = 0; i < IN_FILES; i++)
close(fds[i]);
return 0;
}
//在 select 函数中需要填写参数,如下所示:
res = select(maxfd + 1, &tmp_inset, NULL, NULL, &tv);
相关问题
#include <fcntl.h> #include <unistd> #include <sys/ioctl> #include <sys/time>qt里面定义出错
#include <fcntl.h>是C/C++中的一个头文件,定义了一些文件控制相关的宏和函数。例如,open()函数就是在这个头文件中定义的。在使用这个头文件时,需要注意一些编译器的不同,可能会导致一些定义出现错误。同时,<unistd.h>和<sys/ioctl.h>也是一些常用的系统调用头文件。而<sys/types.h>、<sys/socket.h>、<netdb.h>、<stdio.h>、<stdlib.h>、<string.h>、<ctype.h>、<errno.h>、<malloc.h>、<netinet/in.h>、<arpa/inet.h>、<stdarg.h>等头文件也都是C/C++中常用的头文件,定义了很多系统调用函数和宏。
关于在Qt中出现include头文件定义错误,可能是因为Qt中的一些宏与C/C++中常用的宏冲突了,需要进行一些修改和调整。
使用#include <linux/keyboard.h> 实现截屏键控制
在Linux系统中,可以使用`<linux/keyboard.h>`头文件中的函数来实现截屏键控制。一般步骤如下:
1. 打开键盘设备:使用`open()`函数打开键盘设备文件,以便读取键盘事件。
2. 监听键盘事件:使用`ioctl()`函数设置键盘设备的工作模式,以便在截屏键被按下时进行响应。
3. 截屏操作:当截屏键被按下时,使用Linux Framebuffer或者X11等库进行截屏操作,将屏幕内容保存到文件或内存中。
4. 控制截屏操作:在截屏操作之前,可以进行一些控制,例如确定截屏区域、截屏格式等。
5. 处理截屏结果:截屏操作完成后,可以对截屏结果进行处理,例如显示在窗口中、保存到文件中等。
下面是一个简单的示例,演示如何使用Linux系统的`<linux/keyboard.h>`头文件实现截屏键控制:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <fcntl.h>
#include <linux/input.h>
#define KEY_PRT_SCR 99
int main()
{
int fd, size;
struct input_event ev;
// 打开键盘设备文件
fd = open("/dev/input/event0", O_RDONLY);
if (fd < 0) {
printf("Failed to open device file.\n");
return -1;
}
// 设置键盘设备的工作模式
ioctl(fd, EVIOCGRAB, 1);
while (1) {
// 读取键盘事件
size = read(fd, &ev, sizeof(struct input_event));
if (size < sizeof(struct input_event)) {
printf("Failed to read keyboard event.\n");
close(fd);
return -1;
}
// 按下截屏键时进行截屏操作
if (ev.type == EV_KEY && ev.code == KEY_PRT_SCR && ev.value == 1) {
// TODO: 进行截屏操作
printf("Screen captured.\n");
}
}
// 关闭键盘设备文件
close(fd);
return 0;
}
```
在上面的示例中,我们使用`<linux/input.h>`头文件中的结构体`input_event`来读取键盘事件。当截屏键(`KEY_PRT_SCR`)被按下时,可以进行截屏操作。注意,这里使用了`EVIOCGRAB`命令来设置键盘设备的工作模式,以便在程序运行期间独占键盘设备。
阅读全文
相关推荐
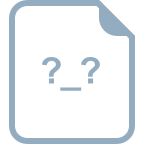
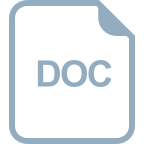
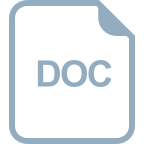













