本关的编程任务是补全右侧代码片段中Begin至End中间的代码,具体要求如下: 往串口里面写数据; 将串口中的数据读取出来。 本关涉及的代码文件UartRWTest.c的代码框架如下: #include <stdio.h> #include <stdlib.h> #include <string.h> #include <unistd.h> /*需要写入的数据的指针为wbuf,存放读取到底数据的缓冲区为rbuf,并将读到的数据长度作为函数返回值*/ int uart_rw(int fd, const char *wbuf, char *rbuf) { /********Begin********/ /*********End*********/ }
时间: 2023-06-29 12:13:29 浏览: 181
下面是补全后的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <fcntl.h>
#include <termios.h>
int uart_rw(int fd, const char *wbuf, char *rbuf) {
int ret = 0;
fd_set read_fds;
struct timeval timeout;
int len = strlen(wbuf);
// 发送数据
ret = write(fd, wbuf, len);
if (ret < 0) {
perror("write");
return -1;
}
// 接收数据
FD_ZERO(&read_fds);
FD_SET(fd, &read_fds);
timeout.tv_sec = 5; // 设置超时时间为5秒
timeout.tv_usec = 0;
ret = select(fd + 1, &read_fds, NULL, NULL, &timeout);
if (ret < 0) {
perror("select");
return -1;
} else if (ret == 0) {
printf("uart_rw: timeout\n");
return -1;
} else {
ret = read(fd, rbuf, 1024);
if (ret < 0) {
perror("read");
return -1;
}
rbuf[ret] = 0;
}
return ret;
}
```
以上代码中,`uart_rw()` 函数用于往串口里面写数据,并将串口中的数据读取出来。具体实现步骤如下:
1. 使用 `write()` 函数向串口写入数据;
2. 使用 `select()` 函数监听串口是否有数据可读,设置超时时间为5秒;
3. 如果 `select()` 函数返回值小于0,则表示出错,直接返回 -1;
4. 如果 `select()` 函数返回值为0,则表示超时,直接返回 -1;
5. 如果 `select()` 函数返回值大于0,则表示串口中有数据可读,使用 `read()` 函数读取数据,并将读取到的数据存放在 `rbuf` 缓冲区中;
6. 如果 `read()` 函数返回值小于0,则表示出错,直接返回 -1;
7. 如果 `read()` 函数返回值大于等于0,则表示读取成功,将读取到的数据长度作为函数返回值返回。
需要注意的是,以上代码中使用了异步I/O模型,即当串口中没有数据可读时,`select()` 函数会阻塞程序,直到串口中有数据可读或者超时时间到。
相关推荐
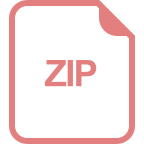
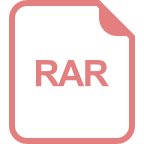
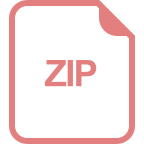














