我需要基于A星算法求解多机器人栅格路径规划的python代码
时间: 2023-10-03 09:02:20 浏览: 39
好的,以下是一个简单的基于A星算法的多机器人栅格路径规划Python代码示例:
```python
import heapq
class Robot:
def __init__(self, start, goal):
self.start = start
self.goal = goal
class Grid:
def __init__(self, width, height, obstacles, robots):
self.width = width
self.height = height
self.obstacles = obstacles
self.robots = robots
def is_obstacle(self, x, y):
return (x, y) in self.obstacles
def is_goal(self, x, y):
for robot in self.robots:
if robot.goal == (x, y):
return True
return False
def cost(self, x1, y1, x2, y2):
return 1
class Node:
def __init__(self, x, y, g, h, parent):
self.x = x
self.y = y
self.g = g
self.h = h
self.parent = parent
def f(self):
return self.g + self.h
def a_star(grid, robots):
start_nodes = [Node(robot.start[0], robot.start[1], 0, 0, None) for robot in robots]
open_set = []
closed_set = set()
for start_node in start_nodes:
heapq.heappush(open_set, (start_node.f(), start_node))
while open_set:
_, current_node = heapq.heappop(open_set)
if all(grid.is_goal(current_node.x, current_node.y) for robot in robots):
paths = []
for i, robot in enumerate(robots):
path = []
node = current_node
while node:
path.append((node.x, node.y))
node = node.parent[i]
paths.append(path[::-1])
return paths
closed_set.add((current_node.x, current_node.y))
for dx, dy in [(0, 1), (0, -1), (1, 0), (-1, 0)]:
x = current_node.x + dx
y = current_node.y + dy
g = current_node.g + grid.cost(current_node.x, current_node.y, x, y)
h = sum(manhattan_distance(x, y, robot.goal[0], robot.goal[1]) for robot in robots)
node = Node(x, y, g, h, current_node)
if x < 0 or x >= grid.width or y < 0 or y >= grid.height \
or grid.is_obstacle(x, y) or (x, y) in closed_set:
continue
heapq.heappush(open_set, (node.f(), node))
return None
def manhattan_distance(x1, y1, x2, y2):
return abs(x1 - x2) + abs(y1 - y2)
# 示例用法
obstacles = [(1, 2), (2, 2), (3, 2), (4, 2), (5, 2), (6, 2)]
robots = [Robot((1, 1), (6, 5)), Robot((1, 3), (6, 1))]
grid = Grid(7, 6, obstacles, robots)
paths = a_star(grid, robots)
print(paths)
```
其中,`Robot` 类表示一个机器人,包含起点和终点坐标;`Grid` 类表示栅格地图,包含宽度、高度、障碍物坐标和机器人列表;`Node` 类表示 A* 算法中的节点,包含坐标、从起点到该点的代价、估价函数值和父节点。`a_star` 函数使用 A* 算法求解多机器人栅格路径规划问题,返回每个机器人的路径。`manhattan_distance` 函数计算两点之间的曼哈顿距离。在示例中,定义了一个 7x6 的栅格地图,其中包含了一些障碍物和两个机器人,调用 `a_star` 函数求解路径并输出结果。
相关推荐
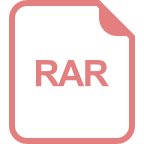














