写出一个基于lstm的多机器人路径规划的python代码,结果用栅格地图中多条路径表示
时间: 2024-05-07 19:21:17 浏览: 11
首先,需要准备一个栅格地图,可以使用numpy数组表示。其中,0表示障碍物,1表示可以通过的空间。假设地图大小为10x10,代码如下:
```python
import numpy as np
map_size = 10
obstacle_ratio = 0.3
obstacle_map = np.random.choice([0, 1], size=(map_size, map_size), p=[obstacle_ratio, 1-obstacle_ratio])
```
接下来,定义一个LSTM模型,用于路径规划。我们使用Keras框架来搭建模型。模型的输入是当前机器人的位置和目标位置,输出是下一步机器人应该移动的方向。代码如下:
```python
from keras.models import Sequential
from keras.layers import Dense, LSTM
input_dim = 2
output_dim = 4
hidden_dim = 8
model = Sequential()
model.add(LSTM(hidden_dim, input_dim=input_dim, return_sequences=True))
model.add(LSTM(hidden_dim))
model.add(Dense(output_dim, activation='softmax'))
model.compile(loss='categorical_crossentropy', optimizer='adam', metrics=['accuracy'])
```
接下来,定义一个多机器人路径规划的函数,用于生成多条路径。该函数接受机器人数量和起点、终点坐标作为输入,返回一组路径。代码如下:
```python
def multi_robot_path_planning(num_robots, start_coords, end_coords, obstacle_map):
paths = []
for i in range(num_robots):
path = []
current_coords = start_coords[i]
while current_coords != end_coords[i]:
# 根据当前机器人的位置和目标位置,计算下一步机器人应该移动的方向
input_data = np.array([current_coords, end_coords[i]])
output_data = model.predict(input_data)
movement_direction = np.argmax(output_data)
# 根据方向更新机器人的位置
if movement_direction == 0:
current_coords = [current_coords[0]-1, current_coords[1]]
elif movement_direction == 1:
current_coords = [current_coords[0]+1, current_coords[1]]
elif movement_direction == 2:
current_coords = [current_coords[0], current_coords[1]-1]
else:
current_coords = [current_coords[0], current_coords[1]+1]
# 将更新后的机器人位置加入路径中
path.append(current_coords)
paths.append(path)
return paths
```
最后,我们可以使用matplotlib库将生成的路径可视化。代码如下:
```python
import matplotlib.pyplot as plt
def plot_paths(paths, obstacle_map):
plt.imshow(obstacle_map, cmap='gray')
for path in paths:
path = np.array(path)
plt.plot(path[:, 1], path[:, 0], linewidth=2)
plt.show()
start_coords = [[0, 0], [1, 1], [2, 2]]
end_coords = [[9, 9], [8, 8], [7, 7]]
paths = multi_robot_path_planning(3, start_coords, end_coords, obstacle_map)
plot_paths(paths, obstacle_map)
```
相关推荐
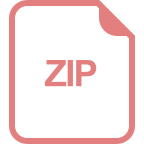
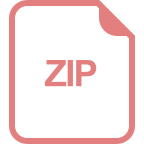














