用C语言递归算法实现二分查找
时间: 2023-07-19 22:14:44 浏览: 65
好的,下面是用C语言递归算法实现二分查找的代码:
```c
#include <stdio.h>
int binarySearch(int arr[], int left, int right, int target) {
if (right >= left) {
int mid = left + (right - left) / 2;
if (arr[mid] == target) {
return mid;
}
if (arr[mid] > target) {
return binarySearch(arr, left, mid - 1, target);
}
return binarySearch(arr, mid + 1, right, target);
}
return -1;
}
int main() {
int arr[] = {2, 4, 6, 8, 10, 12, 14, 16};
int n = sizeof(arr) / sizeof(arr[0]);
int target = 10;
int result = binarySearch(arr, 0, n - 1, target);
if (result == -1) {
printf("Element not found");
} else {
printf("Element found at index %d", result);
}
return 0;
}
```
上述代码中,`binarySearch`函数实现了二分查找。它接收一个整数数组、左边界、右边界和目标元素作为参数,并返回目标元素在数组中的索引。如果目标元素不在数组中,则返回-1。
在函数中,我们首先计算中间元素的索引,然后将目标元素与中间元素进行比较。如果中间元素等于目标元素,则返回中间元素的索引。如果中间元素大于目标元素,则递归调用`binarySearch`函数,在左子数组中查找目标元素。如果中间元素小于目标元素,则递归调用`binarySearch`函数,在右子数组中查找目标元素。
在`main`函数中,我们定义了一个整数数组`arr`、目标元素`target`,并调用`binarySearch`函数在数组中查找目标元素。最后,根据返回值判断目标元素是否在数组中,并输出结果。
相关推荐
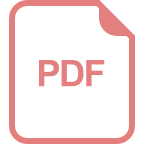
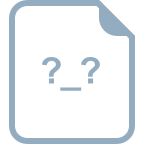














