python用和风天气API获取辽宁省各个市的近几天的天气并导出到excel
时间: 2024-06-09 15:06:51 浏览: 107
好的,首先你需要注册和风天气的开发者账号,然后创建一个应用并获取到你的API Key。接下来你需要安装requests和openpyxl这两个库,你可以在终端中使用以下命令安装:
```
pip install requests
pip install openpyxl
```
然后,你可以使用以下代码获取辽宁省各个市的近几天的天气并导出到excel:
```python
import requests
import openpyxl
# 和风天气API的url
url = "https://devapi.qweather.com/v7/weather/3d"
# 填入你的API Key
key = "your_api_key"
# 辽宁省各个市的城市代码
city_codes = {
"沈阳": "101070101",
"大连": "101070201",
"鞍山": "101070301",
"抚顺": "101070401",
"本溪": "101070501",
"丹东": "101070601",
"锦州": "101070701",
"营口": "101070801",
"阜新": "101070901",
"辽阳": "101071001",
"盘锦": "101071301",
"铁岭": "101071101",
"朝阳": "101071201"
}
# 创建一个新的excel文件
workbook = openpyxl.Workbook()
# 遍历每个城市
for city, code in city_codes.items():
# 构造请求参数
params = {
"key": key,
"location": code
}
# 发送请求并获取响应
response = requests.get(url, params=params)
data = response.json()
# 获取近三天的天气信息
daily_forecasts = data["daily"]
forecasts = []
for forecast in daily_forecasts:
date = forecast["fxDate"]
text_day = forecast["textDay"]
text_night = forecast["textNight"]
temp_max = forecast["tempMax"]
temp_min = forecast["tempMin"]
wind_dir = forecast["windDirDay"]
wind_scale = forecast["windScaleDay"]
forecasts.append([date, text_day, text_night, temp_max, temp_min, wind_dir, wind_scale])
# 将天气信息写入excel文件
worksheet = workbook.create_sheet(city)
worksheet.append(["日期", "白天天气", "晚上天气", "最高温度", "最低温度", "风向", "风力"])
for forecast in forecasts:
worksheet.append(forecast)
# 保存excel文件
workbook.save("weather.xlsx")
```
这段代码会在当前目录下创建一个名为`weather.xlsx`的excel文件,并在每个城市的sheet中写入近三天的天气信息。请注意将代码中的`your_api_key`替换为你自己的和风天气API Key。
阅读全文
相关推荐
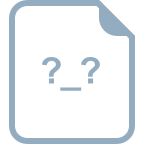

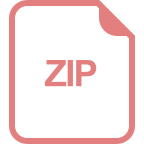
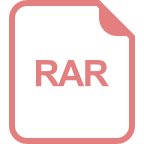
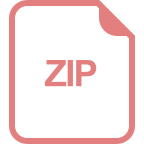
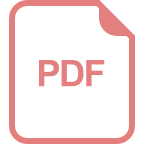
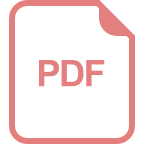
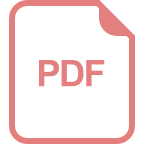
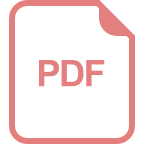
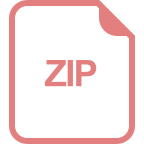
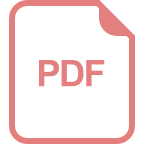
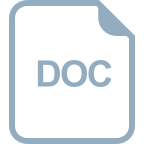
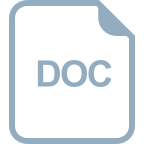