def accuracy(self, y: np.ndarray, prob: np.ndarray)-> float: ''' compute accuracy Parameters: y is the true label. y is a one dimensional array. prob is the predicted label probability. prob is a one dimensional array. Return: acc is the accuracy value ''' #### write your code below #### #### write your code above ####。请帮我完成需要填写的代码
时间: 2024-03-27 15:34:52 浏览: 46
好的,根据您提供的代码,以下是需要填写的代码:
```python
# compute accuracy
pred_label = (prob >= 0.5).astype(int)
acc = np.mean(pred_label == y)
return acc
```
以上代码会将模型预测的概率值转换为标签,规定概率大于等于 0.5 的为正类(1),小于 0.5 的为负类(0),然后计算分类的准确率。
希望对您有帮助!
相关问题
mnist = tf.keras.datasets.mnist.load_data() train_img = mnist[0][0] # 表示训练集 构成的矩阵 表示的 图片 test_img = mnist[1][0] # 表示测试集 构成的矩阵 表示的 图片 train_label = mnist[0][1] # 表示训练集 的图片 表示的 数字是多少 test_label = mnist[1][1] with tf.Session() as sess: init = tf.global_variables_initializer() sess.run(init) start = time.time() for i in range(max_epoch): batch_xs, batch_ys = train_img.next_batch(100) # 此batch是个2维tuple,batch[0]是(100,784)的样本数据数组,batch[1]是(100,10)的样本标签数组 sess.run(train_step, feed_dict={xs: batch_xs, ys: batch_ys, keep_prob: keep_prob_rate}) if (i+1) % 50 == 0: print("step %d, test accuracy %g" % ((i+1), compute_accuracy( mnist.test_images, mnist.test_labels))) end = time.time() print('******************************************************') print("运行时间:%.2f秒" % (end - start)) 出现AttributeError: 'numpy.ndarray' object has no attribute 'next_batch'
根据你的代码,你尝试在 `train_img` 上调用 `next_batch` 属性,但是出现了 `AttributeError: 'numpy.ndarray' object has no attribute 'next_batch'` 的错误。
这个错误是因为 `train_img` 是一个 NumPy 数组对象,而 NumPy 数组没有名为 `next_batch` 的属性。根据你的代码逻辑,你可能想要使用 TensorFlow 中的数据集对象(例如 `tf.data.Dataset`)来获取批次数据。
你可以使用 `tf.data.Dataset.from_tensor_slices` 方法来创建一个数据集对象,并使用 `batch` 方法来获取批次数据。以下是一个示例:
```python
import tensorflow as tf
mnist = tf.keras.datasets.mnist.load_data()
train_img = mnist[0][0] # 表示训练集 构成的矩阵 表示的 图片
train_label = mnist[0][1] # 表示训练集 的图片 表示的 数字是多少
# 创建数据集对象
dataset = tf.data.Dataset.from_tensor_slices((train_img, train_label))
# 对数据集进行乱序处理和批次化处理
dataset = dataset.shuffle(buffer_size=10000).batch(100)
# 创建迭代器
iterator = dataset.make_initializable_iterator()
next_batch = iterator.get_next()
with tf.Session() as sess:
init = tf.global_variables_initializer()
sess.run(init)
sess.run(iterator.initializer) # 初始化迭代器
start = time.time()
for i in range(max_epoch):
batch_xs, batch_ys = sess.run(next_batch)
sess.run(train_step, feed_dict={xs: batch_xs, ys: batch_ys, keep_prob: keep_prob_rate})
if (i+1) % 50 == 0:
print("step %d, test accuracy %g" % ((i+1), compute_accuracy(
mnist.test_images, mnist.test_labels)))
end = time.time()
print('******************************************************')
print("运行时间:%.2f秒" % (end - start))
```
这样,你就可以正确地获取批次数据并进行训练了。请确保你已经正确地定义了 `xs`、`ys`、`train_step`、`compute_accuracy` 等变量。如果还有其他问题,请提供更多的上下文或完整的代码,以便我能够更好地帮助你。
with tf.Session() as sess: init = tf.global_variables_initializer() sess.run(init) sess.run(iterator.initializer) # 初始化迭代器 print("step 0, test accuracy %g" % compute_accuracy(test_img, test_label)) start = time.time() for i in range(max_epoch): batch_xs, batch_ys = sess.run(next_batch) # 此batch是个2维tuple,batch[0]是(100,784)的样本数据数组,batch[1]是(100,10)的样本标签数组 sess.run(train_step, feed_dict={xs: batch_xs, ys: batch_ys, keep_prob: keep_prob_rate}) if (i+1) % 50 == 0: print("step %d, test accuracy %g" % ((i+1), compute_accuracy(mnist.test_images, mnist.test_labels))) end = time.time() print('******************************************************') print("运行时间:%.2f秒" % (end - start)) 出现Traceback (most recent call last): File "D:\PycharmProjects\CNN_mnist\lab.py", line 104, in <module> print("step 0, test accuracy %g" % compute_accuracy(test_img, test_label)) File "D:\PycharmProjects\CNN_mnist\lab.py", line 46, in compute_accuracy y_pre = sess.run(prediction, feed_dict={xs: v_xs, keep_prob: 1}) File "D:\Anaconda3\envs\tf26gpu\lib\site-packages\tensorflow\python\client\session.py", line 967, in run result = self._run(None, fetches, feed_dict, options_ptr, File "D:\Anaconda3\envs\tf26gpu\lib\site-packages\tensorflow\python\client\session.py", line 1164, in _run raise ValueError( ValueError: Cannot feed value of shape (10000, 28, 28) for Tensor 'x_input:0', which has shape '(?, 784)'
根据你提供的错误信息,出现了一个 `ValueError`。错误信息显示无法将形状为 `(10000, 28, 28)` 的值传递给形状为 `(?, 784)` 的张量 `x_input`。
这个错误是因为你的输入数据 `test_img` 的形状是 `(10000, 28, 28)`,但是模型的输入张量 `x_input` 的形状应该是 `(?, 784)`,其中 `?` 表示批次大小。这意味着你需要对输入数据进行扁平化处理,将其从三维的形状 `(10000, 28, 28)` 转换为二维的形状 `(10000, 784)`。
你可以使用 `reshape` 方法来实现这个操作。修改代码如下:
```python
test_img = test_img.reshape(-1, 784) # 将测试集图片数据扁平化
with tf.Session() as sess:
init = tf.global_variables_initializer()
sess.run(init)
sess.run(iterator.initializer) # 初始化迭代器
# 在训练之前先计算初始的测试准确率
print("step 0, test accuracy %g" % compute_accuracy(test_img, test_label))
start = time.time()
for i in range(max_epoch):
batch_xs, batch_ys = sess.run(next_batch)
sess.run(train_step, feed_dict={xs: batch_xs, ys: batch_ys, keep_prob: keep_prob_rate})
if (i+1) % 50 == 0:
# 在每个训练步骤后计算并打印测试准确率
print("step %d, test accuracy %g" % ((i+1), compute_accuracy(test_img, test_label)))
end = time.time()
print('******************************************************')
print("运行时间:%.2f秒" % (end - start))
```
这样,你的输入数据的形状就与模型的输入张量匹配了。如果还有其他问题,请提供更多的上下文或完整的代码,以便我能够更好地帮助你。
阅读全文
相关推荐
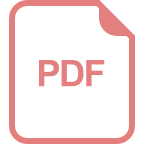
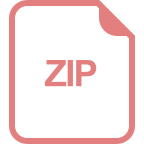
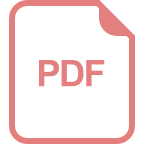
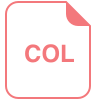
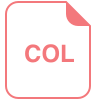
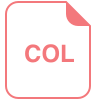
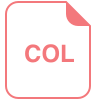
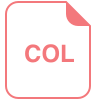
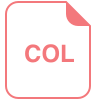
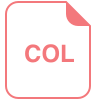






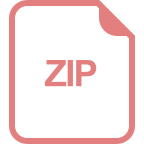
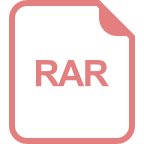