怎么判断文本文件是否为UTF-8编码,用C++代码实现
时间: 2023-07-15 07:14:54 浏览: 96
判断文本文件是否为UTF-8编码,可以通过一些特定的规则来实现,下面是一个基本的C++代码示例:
```c++
#include <fstream>
#include <iostream>
bool is_utf8(const std::string& filename) {
std::ifstream file(filename, std::ios::binary);
if (!file.is_open()) {
return false;
}
char c;
while (file.get(c)) {
if ((c & 0x80) == 0x00) { // ASCII字符,单字节
continue;
} else if ((c & 0xE0) == 0xC0) { // 双字节UTF-8字符
if (!(file.get(c) && (c & 0xC0) == 0x80)) {
return false;
}
} else if ((c & 0xF0) == 0xE0) { // 三字节UTF-8字符
if (!(file.get(c) && (c & 0xC0) == 0x80 && file.get(c) && (c & 0xC0) == 0x80)) {
return false;
}
} else if ((c & 0xF8) == 0xF0) { // 四字节UTF-8字符
if (!(file.get(c) && (c & 0xC0) == 0x80 && file.get(c) && (c & 0xC0) == 0x80 && file.get(c) && (c & 0xC0) == 0x80)) {
return false;
}
} else {
return false;
}
}
return true;
}
int main() {
std::string filename = "test.txt";
bool result = is_utf8(filename);
if (result) {
std::cout << "文件 " << filename << " 是UTF-8编码" << std::endl;
} else {
std::cout << "文件 " << filename << " 不是UTF-8编码" << std::endl;
}
return 0;
}
```
该示例代码中,首先打开文件,并以二进制模式读取文件,然后遍历文件中每个字符,并根据UTF-8编码规则进行判断,最后返回判断结果。
相关推荐
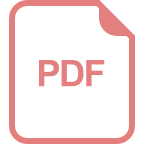
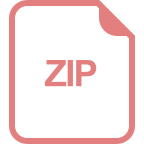
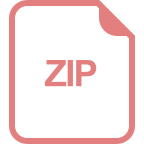














