typescript生成随机数
时间: 2023-09-24 10:13:46 浏览: 349
以下是在typescript中生成随机数的几种方法:
1. 使用Math.random()方法生成0到1之间的随机数,并将其乘以所需的范围(例如100),然后使用Math.floor()方法将结果向下取整,以获得整数随机数。
```typescript
const randomNum = Math.floor(Math.random() * 100); // 生成0到99之间的随机整数
```
2. 使用第三方库(例如lodash)中的函数来生成随机数。例如,使用lodash中的random()方法生成指定范围内的随机数。
```typescript
import { random } from 'lodash';
const randomNum = random(1, 10); // 生成1到10之间的随机数
```
3. 使用crypto模块中的randomBytes()方法生成随机数。此方法生成Buffer对象,因此需要将其转换为所需的格式(例如字符串或数字)。
```typescript
import { randomBytes } from 'crypto';
const randomBuffer = randomBytes(4); // 生成4字节的随机数
const randomNum = parseInt(randomBuffer.toString('hex'), 16); // 将Buffer对象转换为16进制字符串,然后将其转换为数字
```
相关问题
typescript如何生成随机数
TypeScript中可以使用Math.random()方法生成随机数。该方法返回一个0到1之间的随机数,可以通过一些计算来得到不同范围内的随机数。
例如,生成一个0到10之间的随机整数,可以使用以下代码:
```
const randomNum = Math.floor(Math.random() * 11); // 生成0到10的随机整数
```
其中Math.floor()方法用于向下取整,确保生成的随机数为整数。
typescript 生成docx文件
TypeScript可以通过使用适当的库来生成docx文件。其中一个流行的库是"docxtemplater"。这个库允许我们使用模板引擎来生成docx文件。
使用docxtemplater库首先要安装它,通过运行命令`npm install docxtemplater`即可。
然后我们可以在TypeScript中使用这个库来生成docx文件。下面是一个生成docx文件的示例代码:
```typescript
import * as Docxtemplater from 'docxtemplater';
import * as fs from 'fs';
// 读取docx模板文件
const templateContent = fs.readFileSync('template.docx', 'binary');
// 初始化docxtemplater
const doc = new Docxtemplater(templateContent);
// 定义要替换的变量和其对应的值
const data = {
name: 'John Doe',
age: 30,
email: 'johndoe@example.com'
};
// 将数据应用到模板中
doc.setData(data);
// 渲染模板
doc.render();
// 将渲染后的docx文件内容输出到新文件
const output = doc.getZip().generate({
type: 'nodebuffer'
});
fs.writeFileSync('output.docx', output);
```
上述代码首先读取docx模板文件,然后根据定义的变量和对应的值,将数据应用到模板中并渲染模板。最后将渲染后的docx文件内容输出到新的文件中。
这样就可以通过TypeScript生成docx文件了。
相关推荐
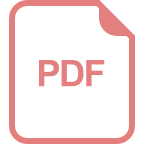
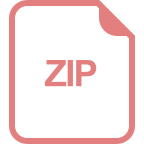










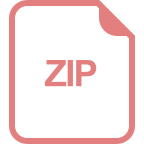
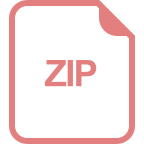