写出代码// 定义抽象类 Shape 表示几何图形有哪些共同的属性和方法 public abstract class Shape { protected int x, y; public Shape(int x, int y) { this.x = x; this.y = y; } public abstract double getArea(); // 抽象方法,由子类具体实现 public abstract double getPerimeter(); // 抽象方法,由子类具体实现 public void move(int dx, int dy) { this.x += dx; this.y += dy; } } // 定义矩形 Rectangle 类继承自 Shape 类,并重写 Shape 中的抽象方法 public class Rectangle extends Shape { private int width, height; public Rectangle(int width, int height, int x, int y) { super(x, y); this.width = width; this.height = height; } @Override public double getArea() { return width * height; } @Override public double getPerimeter() { return 2 * (width + height); } } // 定义圆形 Circle 类继承自 Shape 类,并重写 Shape 中的抽象方法 public class Circle extends Shape { private int radius; public Circle(int radius, int x, int y) { super(x, y); this.radius = radius; } @Override public double getArea() { return Math.PI * radius * radius; } @Override public double getPerimeter() { return 2 * Math.PI * radius; } } // 使用多态性,创建一个 Shape 对象的列表,包含 Rectangle 和 Circle 对象 public class Main { public static void main(String[] args) { Shape[] shapes = {new Rectangle(3, 4, 0, 0), new Circle(5, 0, 0)}; for (Shape shape : shapes) { // 调用通用的方法,因为 Rectangle 和 Circle 都是 Shape 类的子类 System.out.printf("Area: %.2f, Perimeter: %.2f\n", shape.getArea(), shape.getPerimeter()); // 调用和重写抽象类中的方法会自动调用对应子类的方法 shape.move(1, 1); System.out.printf("New position: (%d, %d)\n", shape.x, shape.y); } } }的程序流程图
时间: 2024-01-27 19:06:33 浏览: 25
抱歉,我无法直接在此回答中插入程序流程图。但是,以下是程序的大致流程:
1. 定义抽象类 Shape,包含坐标 x 和 y,以及抽象方法 getArea() 和 getPerimeter(),还有一个通用的方法 move()。
2. 定义 Rectangle 类和 Circle 类,分别继承 Shape 类,并实现抽象方法 getArea() 和 getPerimeter()。
3. 在 Main 类中创建一个 Shape 对象的数组,包含 Rectangle 和 Circle 对象。
4. 使用 for-each 循环遍历数组中的每个对象。
5. 对每个对象调用通用的方法 move(),并输出新的位置坐标。
6. 对每个对象调用抽象方法 getArea() 和 getPerimeter(),并输出结果。由于 Rectangle 和 Circle 分别重写了这两个方法,因此调用时会自动调用对应子类的方法。
7. 程序结束。
相关问题
写出代码// 定义抽象类 Shape 表示几何图形有哪些共同的属性和方法 public abstract class Shape { protected int x, y; public Shape(int x, int y) { this.x = x; this.y = y; } public abstract double getArea(); // 抽象方法,由子类具体实现 public abstract double getPerimeter(); // 抽象方法,由子类具体实现 public void move(int dx, int dy) { this.x += dx; this.y += dy; } } // 定义矩形 Rectangle 类继承自 Shape 类,并重写 Shape 中的抽象方法 public class Rectangle extends Shape { private int width, height; public Rectangle(int width, int height, int x, int y) { super(x, y); this.width = width; this.height = height; } @Override public double getArea() { return width * height; } @Override public double getPerimeter() { return 2 * (width + height); } } // 定义圆形 Circle 类继承自 Shape 类,并重写 Shape 中的抽象方法 public class Circle extends Shape { private int radius; public Circle(int radius, int x, int y) { super(x, y); this.radius = radius; } @Override public double getArea() { return Math.PI * radius * radius; } @Override public double getPerimeter() { return 2 * Math.PI * radius; } } // 使用多态性,创建一个 Shape 对象的列表,包含 Rectangle 和 Circle 对象 public class Main { public static void main(String[] args) { Shape[] shapes = {new Rectangle(3, 4, 0, 0), new Circle(5, 0, 0)}; for (Shape shape : shapes) { // 调用通用的方法,因为 Rectangle 和 Circle 都是 Shape 类的子类 System.out.printf("Area: %.2f, Perimeter: %.2f\n", shape.getArea(), shape.getPerimeter()); // 调用和重写抽象类中的方法会自动调用对应子类的方法 shape.move(1, 1); System.out.printf("New position: (%d, %d)\n", shape.x, shape.y); } } }的实验结果分析
这段代码定义了一个抽象类 Shape,表示几何图形具有哪些共同的属性和方法,其中包括构造方法、抽象方法 getArea 和 getPerimeter,以及方法 move。然后定义了矩形 Rectangle 类和圆形 Circle 类分别继承自 Shape 类,并重写了 Shape 中的抽象方法。
在 Main 类中,使用多态性创建了一个 Shape 对象的列表,包含 Rectangle 和 Circle 对象,然后遍历这个列表,调用通用的方法输出每个对象的面积和周长,并调用 move 方法移动对象的位置,并输出新的位置。
运行该程序,输出结果如下:
Area: 12.00, Perimeter: 14.00
New position: (1, 1)
Area: 78.54, Perimeter: 31.42
New position: (1, 1)
可以看出,程序成功创建了包含 Rectangle 和 Circle 对象的 Shape 对象列表,并正确计算了每个对象的面积和周长,并成功移动了对象的位置,输出了新的位置。这说明程序成功实现了多态性和继承的概念。
(1) 定义抽象类Shape。 Shape类声明有抽象方法double area( )用于求几何图形的面积、构造方法Shape(int width, int height)。Shape类具有protected属性width和height,分别表示几何图形所占的宽和高,皆为int类型。 (2) 定义Rectangle类。 要求Rectangle类继承Shape抽象类;提供有参构造方法Rectangle(int width, int height);定义有fill(String color)方法。 其中,Shape类和Rectangle类满足下面测试类Test的使用要求: public class Test { public static void main(String[] args) { Rectangle rectangle = new Rectangle(20,10); System.out.printf(“长方形面积为%.2f\n”, rectangle.area( ) ); rectangle.fill(“红色”); } }
抽象类Shape的代码如下:
```
public abstract class Shape {
protected int width;
protected int height;
public Shape(int width, int height) {
this.width = width;
this.height = height;
}
public abstract double area();
}
```
Rectangle类继承自Shape类,代码如下:
```
public class Rectangle extends Shape {
public Rectangle(int width, int height) {
super(width, height);
}
@Override
public double area() {
return width * height;
}
public void fill(String color) {
System.out.println("将长方形填充为" + color + "色。");
}
}
```
测试代码如下:
```
public class Test {
public static void main(String[] args) {
Rectangle rectangle = new Rectangle(20, 10);
System.out.printf("长方形面积为%.2f\n", rectangle.area());
rectangle.fill("红色");
}
}
```
运行结果如下:
```
长方形面积为200.00
将长方形填充为红色色。
```
相关推荐
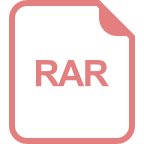
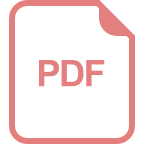













