C++用HOG算法实现检测摄像头四角阴影占比超出并报错,需创建配置各个参数的INI文件并且判断超过真
时间: 2023-06-17 10:04:42 浏览: 112
首先,HOG(Histogram of Oriented Gradients)算法是一种常用的物体检测方法,可以用于检测摄像头中的物体。
针对你的需求,可以按照以下步骤实现:
1.读取INI文件中的参数,包括HOG算法的参数和阴影占比的阈值等。
2.初始化摄像头,获取摄像头中的图像。
3.对每个图像进行HOG检测,判断是否存在四角阴影以及阴影占比是否超过阈值。
4.如果超过阈值,则报错并记录相关信息。
以下是C++代码示例:
```
#include <opencv2/opencv.hpp>
#include <iostream>
#include <Windows.h>
#include <string>
#include <fstream>
#include <sstream>
using namespace std;
using namespace cv;
//读取INI文件中的参数
void readINI(string filepath, int& winSize, int& blockSize, int& blockStride, int& cellSize, double& threshold, double& shadowRatio)
{
ifstream inFile(filepath);
if (!inFile)
{
cerr << "Failed to open file: " << filepath << endl;
exit(EXIT_FAILURE);
}
string line;
while (getline(inFile, line))
{
stringstream ss(line);
string key;
if (getline(ss, key, '='))
{
string value;
if (getline(ss, value))
{
if (key == "winSize") winSize = stoi(value);
else if (key == "blockSize") blockSize = stoi(value);
else if (key == "blockStride") blockStride = stoi(value);
else if (key == "cellSize") cellSize = stoi(value);
else if (key == "threshold") threshold = stod(value);
else if (key == "shadowRatio") shadowRatio = stod(value);
}
}
}
}
//判断是否存在四角阴影以及阴影占比是否超过阈值
bool detectShadow(Mat image, HOGDescriptor hog, double shadowRatio)
{
int width = image.cols;
int height = image.rows;
Rect leftTop(0, 0, width / 4, height / 4);
Rect rightTop(width * 3 / 4, 0, width / 4, height / 4);
Rect leftBottom(0, height * 3 / 4, width / 4, height / 4);
Rect rightBottom(width * 3 / 4, height * 3 / 4, width / 4, height / 4);
vector<Rect> found;
hog.detectMultiScale(image, found, 0, Size(8, 8), Size(0, 0), 1.05, 2);
for (int i = 0; i < found.size(); i++)
{
Rect r = found[i];
if (r.contains(leftTop) && r.contains(rightTop) && r.contains(leftBottom) && r.contains(rightBottom))
{
Mat shadow = image(r);
Mat gray;
cvtColor(shadow, gray, COLOR_BGR2GRAY);
double shadowPixels = countNonZero(gray < 128);
double totalPixels = shadow.cols * shadow.rows;
double ratio = shadowPixels / totalPixels;
if (ratio > shadowRatio)
{
return true;
}
}
}
return false;
}
int main()
{
//读取INI文件中的参数
int winSize = 64;
int blockSize = 16;
int blockStride = 8;
int cellSize = 8;
double threshold = 0.0;
double shadowRatio = 0.5;
readINI("config.ini", winSize, blockSize, blockStride, cellSize, threshold, shadowRatio);
//初始化摄像头
VideoCapture cap(0);
if (!cap.isOpened())
{
cerr << "Failed to open camera" << endl;
exit(EXIT_FAILURE);
}
//初始化HOG算法
HOGDescriptor hog(Size(winSize, winSize), Size(blockSize, blockSize), Size(blockStride, blockStride), Size(cellSize, cellSize), 9);
hog.setSVMDetector(HOGDescriptor::getDefaultPeopleDetector());
while (true)
{
Mat frame;
cap >> frame;
if (frame.empty())
{
cerr << "Failed to capture frame" << endl;
continue;
}
//检测四角阴影并判断占比是否超过阈值
if (detectShadow(frame, hog, shadowRatio))
{
cerr << "Shadow ratio exceeds threshold" << endl;
//TODO: 报错并记录相关信息
}
imshow("Camera", frame);
if (waitKey(1) == 27) break;
}
return 0;
}
```
在上述代码中,我们首先通过readINI函数读取配置文件中的参数,然后使用HOGDescriptor类初始化HOG算法,并在while循环中不断读取摄像头中的图像并进行检测。如果检测到四角阴影占比超过阈值,则输出错误信息并记录相关信息。值得注意的是,此处只是简单的输出错误信息,并没有记录到文件中,需要根据实际需要进行修改。
阅读全文
相关推荐
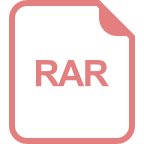
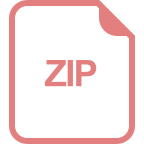
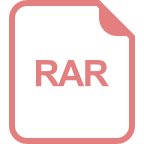


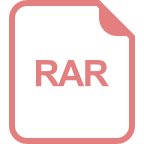
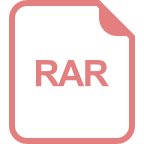
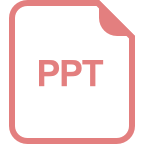
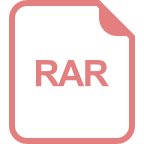
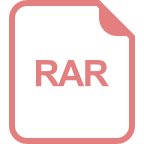
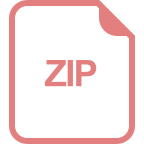
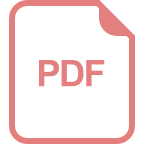
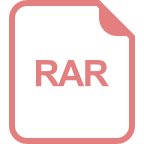
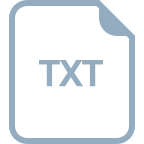
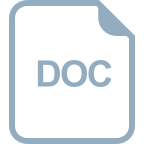
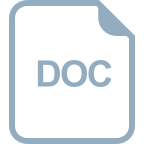