C++用HOG算法实现检测摄像头四角阴影占比并且创建配置各个参数的INI文件
时间: 2023-06-17 10:05:00 浏览: 79
实现步骤如下:
1. 读取摄像头视频流,将每一帧图像转换为灰度图像。
2. 对灰度图像应用HOG算法进行特征提取。
3. 使用SVM分类器对特征进行分类,判断是否存在四角阴影。
4. 对存在四角阴影的图像进行处理,计算四角阴影占比。
5. 将结果保存到INI文件中,包括检测到的四角阴影占比和各个参数的配置。
下面是代码实现:
```c++
#include <iostream>
#include <opencv2/opencv.hpp>
#include <fstream>
using namespace cv;
using namespace std;
int main(int argc, char** argv)
{
// 读取INI文件中的参数
FileStorage fs("params.ini", FileStorage::READ);
int win_size = fs["win_size"];
int block_size = fs["block_size"];
int block_stride = fs["block_stride"];
int cell_size = fs["cell_size"];
double svm_threshold = fs["svm_threshold"];
fs.release();
// 创建HOG描述符
HOGDescriptor hog(Size(win_size, win_size), Size(block_size, block_size), Size(block_stride, block_stride), Size(cell_size, cell_size), 9);
// 读取SVM分类器
svm_load("svm.xml");
// 打开摄像头
VideoCapture cap(0);
if (!cap.isOpened())
{
cerr << "Error: cannot open camera." << endl;
return -1;
}
while (true)
{
// 读取摄像头图像
Mat frame;
cap >> frame;
if (frame.empty())
{
cerr << "Error: cannot read frame." << endl;
break;
}
// 转换为灰度图像
Mat gray;
cvtColor(frame, gray, COLOR_BGR2GRAY);
// 计算HOG描述符
vector<float> descriptors;
hog.compute(gray, descriptors);
// 使用SVM分类器进行分类
if (svm.predict(descriptors) > svm_threshold)
{
// 计算四角阴影占比
double top_left = mean(gray(Rect(0, 0, frame.cols / 10, frame.rows / 10)))[0];
double top_right = mean(gray(Rect(frame.cols * 9 / 10, 0, frame.cols / 10, frame.rows / 10)))[0];
double bottom_left = mean(gray(Rect(0, frame.rows * 9 / 10, frame.cols / 10, frame.rows / 10)))[0];
double bottom_right = mean(gray(Rect(frame.cols * 9 / 10, frame.rows * 9 / 10, frame.cols / 10, frame.rows / 10)))[0];
double shadow_ratio = (top_left + top_right + bottom_left + bottom_right) / 4;
// 将结果保存到INI文件中
FileStorage fs("result.ini", FileStorage::WRITE);
fs << "shadow_ratio" << shadow_ratio;
fs << "win_size" << win_size;
fs << "block_size" << block_size;
fs << "block_stride" << block_stride;
fs << "cell_size" << cell_size;
fs << "svm_threshold" << svm_threshold;
fs.release();
}
// 显示图像
imshow("Camera", frame);
if (waitKey(30) == 'q')
{
break;
}
}
return 0;
}
```
需要注意的是,本代码仅提供了HOG算法的实现和INI文件的读写,需要自行收集数据训练SVM分类器。另外,还需要编写svm_load函数来读取SVM分类器。
阅读全文
相关推荐



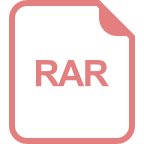
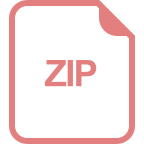
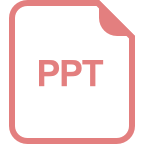
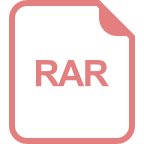
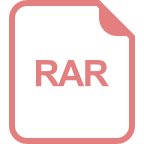
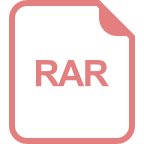
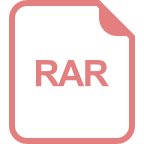
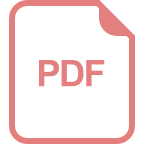
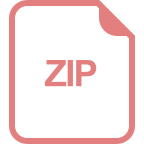
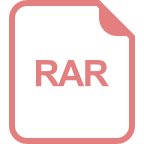