list_num = [] * 100 sum = 0 n,a,b = map(int, input().split()) for i in range(n): list_num.append(input().split(' ')) list_num[i] = list(map(int, list_num[i])) def calculate_sum(n, a, b, sum, list_num): for i in range(n): sum = sum + judge(a, b, list_num[i]) return sum def calculate_w(a, b, list_): if (list_[0] <= 0): if (list_[2] < a): return list_[2] else: return a else: if (list_[2] < a): return list_[2] - list_[0] else: return a - list_[0] def calculate_l(a, b, list_): if (list_[1] <= 0): if (list_[3] < b): return list_[3] else: return b else: if (list_[3] < b): return list_[3] - list_[0] else: return b - list_[0] def calculate(a, b, list_): return calculate_w(a, b, list_) * calculate_l(a, b, list_) def judge(a, b, list_): if (list_[0] > 0 and list_[1] > 0 and list_[2] < a and list_[3] < b): return ((list_[2] - list_[0]) * (list_[3] - list_[1])) elif (list_[0] >= a or list_[1] >= b or list_[2] <= 0 or list_[3] <= 0): return 0 else: return calculate(a, b, list_) if __name__ == '__main__': print(calculate_sum(n, a, b, sum, list_num))转c++
时间: 2024-01-20 21:03:45 浏览: 102
以下是将给定的 Python 代码转换为 C++ 代码的过程:
```c++
#include<iostream>
#include<vector>
#include<algorithm>
using namespace std;
int calculate_w(int a, int b, vector<int>& list_) {
if (list_[0] <= 0) {
if (list_[2] < a) {
return list_[2];
}
else {
return a;
}
}
else {
if (list_[2] < a) {
return list_[2] - list_[0];
}
else {
return a - list_[0];
}
}
}
int calculate_l(int a, int b, vector<int>& list_) {
if (list_[1] <= 0) {
if (list_[3] < b) {
return list_[3];
}
else {
return b;
}
}
else {
if (list_[3] < b) {
return list_[3] - list_[1];
}
else {
return b - list_[1];
}
}
}
int calculate(int a, int b, vector<int>& list_) {
return calculate_w(a, b, list_) * calculate_l(a, b, list_);
}
int judge(int a, int b, vector<int>& list_) {
if (list_[0] > 0 && list_[1] > 0 && list_[2] < a && list_[3] < b) {
return ((list_[2] - list_[0]) * (list_[3] - list_[1]));
}
else if (list_[0] >= a || list_[1] >= b || list_[2] <= 0 || list_[3] <= 0) {
return 0;
}
else {
return calculate(a, b, list_);
}
}
int calculate_sum(int n, int a, int b, int sum, vector<vector<int>>& list_num) {
for (int i = 0; i < n; i++) {
sum = sum + judge(a, b, list_num[i]);
}
return sum;
}
int main() {
int n, a, b, sum = 0;
cin >> n >> a >> b;
vector<vector<int>> list_num(n, vector<int>(4));
for (int i = 0; i < n; i++) {
for (int j = 0; j < 4; j++) {
cin >> list_num[i][j];
}
}
cout << calculate_sum(n, a, b, sum, list_num);
return 0;
}
```
注意,C++ 中需要使用向量(vector)来存储二维数组。同时,C++ 中的数组下标是从 0 开始的,而 Python 中是从 1 开始的。因此在 C++ 中需要将数组下标都减去 1。
阅读全文
相关推荐
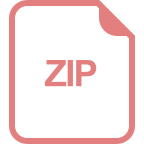



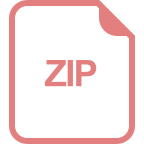











