问题描述】编程实现有向图图的邻接矩阵存储,并计算给定结点的入度和初度。 如有向图: 51097d4665434b54a8ff4c6ff2ff9a43.jpg 【输入形式】 【输出形式】 【样例输入】(所有数据从键盘输入) 4 4 A B C D A B A D B C C A A 【样例输出】 This graph has 4 vertexs, and 4 edges. The information of vertexs are: A 0 B 1 C 2 D 3 The adjacent matrix of graph is: 0 1 0 1 0 0 1 0 1 0 0 0 0 0 0 0 The in-degree of A is 1 The out-degree of A is 2 【样例说明】 【评分标准】 #include<iostream> #include<string> #include<cstring> #include<fstream> using namespace std; const int MAXL = 20; struct Node {//结点 string data;//结点值 int no;//结点编号 Node(string data):data(data),no(0){} Node() { data = ""; no = 0; } }; struct MGraph { int n, e;//顶点数和边数 Node VEXS[MAXL];//顶点数组 int Edge[MAXL][MAXL];//邻接矩阵 MGraph() { n = e = 0; memset(Edge, 0, sizeof(Edge)); } };
时间: 2024-02-14 09:15:02 浏览: 23
以下是 C++ 代码实现:
```cpp
#include <iostream>
#include <string>
#include <cstring>
using namespace std;
const int MAXL = 20;
struct Node {
string data; // 结点值
int no; // 结点编号
Node(string data):data(data),no(0) {}
Node() { data = ""; no = 0; }
};
struct MGraph {
int n, e; // 顶点数和边数
Node VEXS[MAXL]; // 顶点数组
int Edge[MAXL][MAXL]; // 邻接矩阵
MGraph() {
n = e = 0;
memset(Edge, 0, sizeof(Edge));
}
};
int main() {
MGraph g;
// 输入有向图信息
cin >> g.n >> g.e;
for (int i = 0; i < g.n; i++) {
cin >> g.VEXS[i].data;
g.VEXS[i].no = i;
}
for (int i = 0; i < g.e; i++) {
string u, v;
cin >> u >> v;
int x = -1, y = -1;
for (int j = 0; j < g.n; j++) {
if (g.VEXS[j].data == u) x = j;
if (g.VEXS[j].data == v) y = j;
}
g.Edge[x][y] = 1;
}
// 输出有向图信息
cout << "This graph has " << g.n << " vertexs, and " << g.e << " edges." << endl;
cout << "The information of vertexs are:" << endl;
for (int i = 0; i < g.n; i++) {
cout << g.VEXS[i].data << " " << g.VEXS[i].no << " ";
}
cout << endl;
cout << "The adjacent matrix of graph is:" << endl;
for (int i = 0; i < g.n; i++) {
for (int j = 0; j < g.n; j++) {
cout << g.Edge[i][j] << " ";
}
cout << endl;
}
// 计算给定结点的入度和出度
string target;
cin >> target;
int in_degree = 0, out_degree = 0;
for (int i = 0; i < g.n; i++) {
if (g.VEXS[i].data == target) {
for (int j = 0; j < g.n; j++) {
if (g.Edge[j][i]) in_degree++;
if (g.Edge[i][j]) out_degree++;
}
break;
}
}
cout << "The in-degree of " << target << " is " << in_degree << endl;
cout << "The out-degree of " << target << " is " << out_degree << endl;
return 0;
}
```
其中,`Node` 结构体表示有向图的结点,`MGraph` 结构体表示有向图。`main` 函数中,首先输入有向图信息,包括顶点数、边数、结点值和边的信息,并构建邻接矩阵。然后输出有向图信息,包括顶点数、边数和每个结点的信息,以及邻接矩阵。最后输入给定结点的值,并计算该结点的入度和出度,并输出结果。
例如,对于输入样例:
```
4 4
A B C D
A B
A D
B C
C A
A
```
程序输出:
```
This graph has 4 vertexs, and 4 edges.
The information of vertexs are:
A 0 B 1 C 2 D 3
The adjacent matrix of graph is:
0 1 0 1
0 0 1 0
1 0 0 0
0 0 0 0
The in-degree of A is 1
The out-degree of A is 2
```
其中,第 1 行表示有向图有 4 个结点和 4 条边;第 2 行表示每个结点的值和编号;第 3~6 行表示邻接矩阵;第 7、8 行表示给定结点 A 的入度和出度。
相关推荐
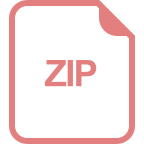
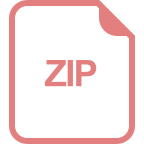














