无向图图的邻接表
时间: 2023-11-05 09:12:13 浏览: 210
无向图的邻接表是一种数据结构,用于表示无向图中每个顶点的邻居顶点。
具体地,对于一个无向图G=(V,E),其中V表示顶点集合,E表示边集合,邻接表的构造方法如下:
1. 对于每个顶点v∈V,创建一个空链表adjList[v],用于存放与v相邻的所有顶点。
2. 遍历边集合E,对于每条边(u,v)∈E,将v加入u的邻接表adjList[u]中,将u加入v的邻接表adjList[v]中。
例如,对于以下无向图:
```
1 -- 2
/ \ |
3 - 4--5
```
其邻接表可以表示为:
```
adjList[1]: 2, 3
adjList[2]: 1, 4, 5
adjList[3]: 1, 4
adjList[4]: 2, 3, 5
adjList[5]: 2, 4
```
其中,adjList[1]表示与顶点1相邻的顶点集合为{2,3},以此类推。
相关问题
无向图图的邻接表实现
邻接表是一种表示图的数据结构,它通过将每个顶点的邻居顶点列表保存在数组中,来描述图的结构。
在无向图中,每个顶点都与其相邻的顶点相连,因此邻接表中的每个顶点都需保存与其相邻的所有顶点。
邻接表的实现可以使用链表或向量(动态数组)等数据结构,其中链表更常用。下面是使用链表实现无向图邻接表的示例代码:
```c++
#include <iostream>
#include <vector>
using namespace std;
// 邻接表的节点
struct Node {
int val; // 顶点的值
Node* next; // 指向下一个节点的指针
Node(int v) : val(v), next(NULL) {}
};
// 无向图邻接表
class Graph {
public:
Graph(int n) : nodes(n), edges(0) {
for (int i = 0; i < n; ++i) {
nodes[i] = NULL;
}
}
// 添加边
void addEdge(int from, int to) {
Node* node = new Node(to);
node->next = nodes[from];
nodes[from] = node;
++edges;
// 由于是无向图,需要添加反向边
node = new Node(from);
node->next = nodes[to];
nodes[to] = node;
++edges;
}
// 获取顶点的所有邻居
vector<int> getNeighbors(int node) {
vector<int> neighbors;
Node* curr = nodes[node];
while (curr) {
neighbors.push_back(curr->val);
curr = curr->next;
}
return neighbors;
}
private:
vector<Node*> nodes; // 存储每个顶点的邻居节点
int edges; // 边数
};
```
上述代码中,Graph 类的构造函数接收顶点的数量,初始化了一个节点数组,每个节点都设置为 NULL。addEdge 函数用于添加边,它向节点数组中的两个顶点添加节点,并将它们互相连接。getNeighbors 函数用于获取一个顶点的所有邻居。
这样,我们就可以使用上述代码来实现无向图的邻接表了。
无向图图的邻接表c++
以下是使用 C++ 实现无向图邻接表的示例代码:
```cpp
#include <iostream>
#include <vector>
using namespace std;
// 定义邻接表的节点结构体
struct GraphNode {
int val; // 节点编号
vector<GraphNode*> neighbors; // 与该节点相邻的其他节点
GraphNode(int x) : val(x) {};
};
// 构造无向图的邻接表
vector<GraphNode*> buildGraph(vector<vector<int>>& edges) {
vector<GraphNode*> graph;
unordered_map<int, GraphNode*> mp;
for (auto& edge : edges) {
int from = edge[0], to = edge[1];
if (!mp.count(from)) {
mp[from] = new GraphNode(from);
}
if (!mp.count(to)) {
mp[to] = new GraphNode(to);
}
mp[from]->neighbors.push_back(mp[to]); // 无向图需要双向连接
mp[to]->neighbors.push_back(mp[from]);
}
for (auto& it : mp) {
graph.push_back(it.second);
}
return graph;
}
int main() {
vector<vector<int>> edges = {{0, 1}, {0, 2}, {0, 3}, {1, 2}, {2, 3}};
vector<GraphNode*> graph = buildGraph(edges);
for (auto& node : graph) {
cout << node->val << ": ";
for (auto& neighbor : node->neighbors) {
cout << neighbor->val << " -> ";
}
cout << "NULL" << endl;
}
return 0;
}
```
以上代码中,我们定义了一个 `GraphNode` 结构体来表示邻接表中的节点,其中包含了节点的编号和与之相邻的其他节点。然后我们使用一个 `unordered_map` 来将每个节点的编号与其对应的结构体对象建立映射关系。接着我们遍历每条边,在 `unordered_map` 中找到对应的节点,将它们之间建立双向连接,最终将所有节点的结构体对象放入一个 vector 中返回。最后遍历这个 vector,打印每个节点的编号以及与之相邻的其他节点的编号即可。
阅读全文
相关推荐
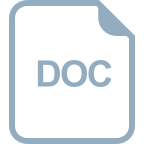

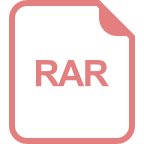
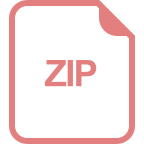
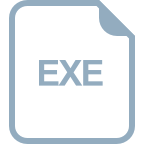
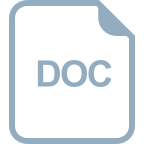




