写个python代码 利用faster_rcnn_resnet50_fpn模型给一张大图和一张小图,从大图中识别小图位置并返回小图在大图中的坐标
时间: 2024-03-04 14:49:40 浏览: 67
好的,以下是一个利用 `faster_rcnn_resnet50_fpn` 模型给一张大图和一张小图,从大图中识别小图位置并返回小图在大图中的坐标的 Python 代码:
```python
import torch
import torchvision
import cv2
import numpy as np
# 加载模型
model = torchvision.models.detection.fasterrcnn_resnet50_fpn(pretrained=True)
# 定义设备,如果有GPU则使用GPU
device = torch.device('cuda' if torch.cuda.is_available() else 'cpu')
# 将模型移动到设备上
model.to(device)
# 设置阈值
threshold = 0.5
# 加载大图和小图
large_image = cv2.imread('large.jpg')
small_image = cv2.imread('small.jpg')
# 转换小图为Tensor,并将其移动到设备上
small_image_tensor = torchvision.transforms.functional.to_tensor(small_image)
small_image_tensor = small_image_tensor.to(device)
# 执行推理
model.eval()
with torch.no_grad():
# 将大图转换为Tensor,并将其移动到设备上
large_image_tensor = torchvision.transforms.functional.to_tensor(large_image)
large_image_tensor = large_image_tensor.to(device)
# 执行推理
outputs = model([large_image_tensor])
# 获取物体框和分数
boxes = outputs[0]['boxes'].cpu().numpy()
scores = outputs[0]['scores'].cpu().numpy()
# 循环遍历所有的物体框
for i in range(len(boxes)):
# 如果物体分数大于阈值,则认为检测到了小图
if scores[i] > threshold:
# 获取物体框的坐标
x1, y1, x2, y2 = boxes[i]
# 将物体框转换为整数类型
x1, y1, x2, y2 = int(x1), int(y1), int(x2), int(y2)
# 从大图中截取出小图
cropped_image = large_image[y1:y2, x1:x2]
# 判断小图和目标小图是否匹配
if cropped_image.shape == small_image.shape:
# 计算小图在大图中的坐标
x, y = x1, y1
# 输出小图在大图中的坐标
print("小图在大图中的坐标为:x={}, y={}".format(x, y))
```
在这个代码中,我们首先加载了 `faster_rcnn_resnet50_fpn` 模型,并将其移动到所选设备(如果有GPU,则使用GPU)。然后,我们加载了大图和小图,并将小图转换为Tensor,并将其移动到设备上。接下来,我们执行推理操作,获取所有的物体框和分数。我们循环遍历所有的物体框,如果物体分数大于阈值,则认为检测到了小图。然后,我们从大图中截取出小图,并计算小图在大图中的坐标。最后,我们输出小图在大图中的坐标。
阅读全文
相关推荐
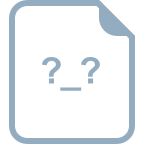

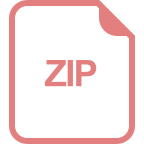
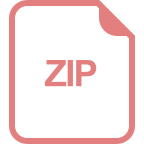
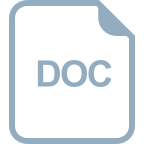




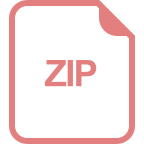
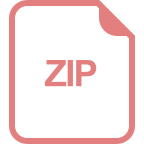
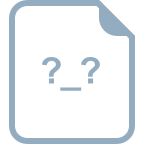
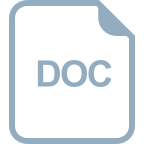
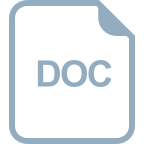


