给出class-agnostic模块作用于faster_RCNN目标检测的pytorch训练示例代码,数据集就定义为COCO数据集,最后给出推理测试代码
时间: 2024-02-18 21:01:40 浏览: 108
以下是class-agnostic模块作用于faster_RCNN目标检测的pytorch训练示例代码,数据集定义为COCO数据集:
```python
import torch
import torchvision
from torchvision.models.detection.faster_rcnn import FastRCNNPredictor
from torchvision.datasets import CocoDetection
from torchvision.transforms import transforms
from torch.utils.data import DataLoader
# define the model
model = torchvision.models.detection.fasterrcnn_resnet50_fpn(pretrained=True)
# get the number of input features for the classifier
in_features = model.roi_heads.box_predictor.cls_score.in_features
# replace the pre-trained head with a new one
model.roi_heads.box_predictor = FastRCNNPredictor(in_features, num_classes=2) # 2 classes: background and object
# define the dataset and data loader
dataset = CocoDetection(root='./coco_dataset', annFile='./coco_dataset/annotations/instances_train2017.json',
transforms=transforms.Compose([transforms.ToTensor()]))
data_loader = DataLoader(dataset, batch_size=2, shuffle=True, num_workers=4, collate_fn=utils.collate_fn)
# define the optimizer and the learning rate scheduler
params = [p for p in model.parameters() if p.requires_grad]
optimizer = torch.optim.SGD(params, lr=0.005, momentum=0.9, weight_decay=0.0005)
lr_scheduler = torch.optim.lr_scheduler.StepLR(optimizer, step_size=3, gamma=0.1)
# train the model for 10 epochs
num_epochs = 10
for epoch in range(num_epochs):
# train for one epoch, printing every 10 iterations
train_one_epoch(model, optimizer, data_loader, device, epoch, print_freq=10)
# update the learning rate
lr_scheduler.step()
# evaluate on the test dataset every epoch
evaluate(model, data_loader_test, device=device)
# save the model
torch.save(model.state_dict(), 'fasterrcnn_resnet50_fpn_class_agnostic.pt')
```
以下是推理测试代码:
```python
import torch
import torchvision
from torchvision.models.detection.faster_rcnn import FastRCNNPredictor
from torchvision.transforms import transforms
import cv2
# define the model
model = torchvision.models.detection.fasterrcnn_resnet50_fpn(pretrained=True)
# get the number of input features for the classifier
in_features = model.roi_heads.box_predictor.cls_score.in_features
# replace the pre-trained head with a new one
model.roi_heads.box_predictor = FastRCNNPredictor(in_features, num_classes=2) # 2 classes: background and object
# load the trained weights
model.load_state_dict(torch.load('fasterrcnn_resnet50_fpn_class_agnostic.pt'))
# set the model to evaluation mode
model.eval()
# define the transforms
transform = transforms.Compose([transforms.ToTensor()])
# load the image
img = cv2.imread('test.jpg')
# apply the transforms
img = transform(img)
# add a batch dimension
img = img.unsqueeze(0)
# forward pass through the model
with torch.no_grad():
predictions = model(img)
# display the results
print(predictions)
```
阅读全文
相关推荐
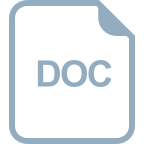
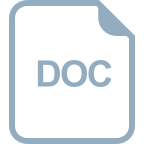
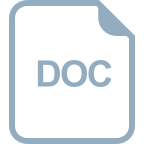





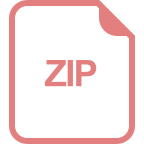
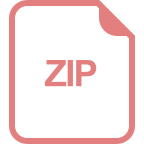
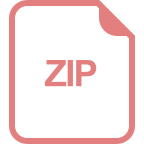







