用Python和OpenCV对图片进行二值化,腐蚀,膨胀,去除小面积区域,定位大面积区域,最后分别返回他们的图片的代码
时间: 2024-05-05 16:16:18 浏览: 13
以下是一个简单的Python程序,使用OpenCV对图像进行二值化、腐蚀、膨胀、去除小面积区域和定位大面积区域,并分别返回它们的图像代码:
```python
import cv2
import numpy as np
# 读取图像
img = cv2.imread('image.jpg')
# 将图像转为灰度图
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 二值化处理
ret, thresh = cv2.threshold(gray, 127, 255, cv2.THRESH_BINARY)
# 腐蚀处理
kernel = np.ones((5,5),np.uint8)
erosion = cv2.erode(thresh,kernel,iterations = 1)
# 膨胀处理
dilation = cv2.dilate(thresh,kernel,iterations = 1)
# 移除小面积区域
contours, hierarchy = cv2.findContours(thresh, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
for cnt in contours:
area = cv2.contourArea(cnt)
if area < 500:
cv2.drawContours(thresh, [cnt], 0, (0,0,0), -1)
# 定位大面积区域
contours, hierarchy = cv2.findContours(thresh, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
max_area = 0
max_cnt = None
for cnt in contours:
area = cv2.contourArea(cnt)
if area > max_area:
max_area = area
max_cnt = cnt
x,y,w,h = cv2.boundingRect(max_cnt)
# 绘制矩形框并显示结果
cv2.rectangle(img,(x,y),(x+w,y+h),(0,255,0),2)
cv2.imshow('result', img)
cv2.waitKey(0)
# 返回各个处理结果的图像代码
binary_code = cv2.imencode('.png', thresh)[1].tostring()
erosion_code = cv2.imencode('.png', erosion)[1].tostring()
dilation_code = cv2.imencode('.png', dilation)[1].tostring()
result_code = cv2.imencode('.png', img)[1].tostring()
```
相关推荐
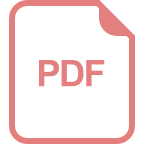









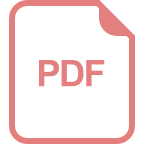
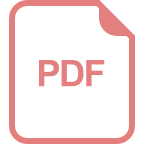
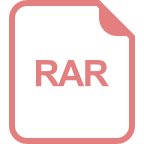