使用光谱重建方法得到光谱响应函数的代码
时间: 2023-07-29 15:08:39 浏览: 267
以下是使用 Python 实现的光谱重建方法代码:
```python
import numpy as np
from scipy.optimize import curve_fit
def gaussian(x, a, b, c):
"""
定义高斯函数
"""
return a * np.exp(-(x - b)**2 / (2 * c**2))
def spectral_response_function(light_source, reflectance, spectrum):
"""
光谱重建方法获取光谱响应函数
"""
# 对反射谱进行归一化处理
reflectance_norm = reflectance / np.max(reflectance)
# 对光源谱进行归一化处理
light_source_norm = light_source / np.max(light_source)
# 对测量谱进行归一化处理
spectrum_norm = spectrum / np.max(spectrum)
# 对反射谱进行平滑处理
fit_params, _ = curve_fit(gaussian, range(len(reflectance_norm)), reflectance_norm)
reflectance_smooth = gaussian(range(len(reflectance_norm)), *fit_params)
# 对光源谱进行平滑处理
fit_params, _ = curve_fit(gaussian, range(len(light_source_norm)), light_source_norm)
light_source_smooth = gaussian(range(len(light_source_norm)), *fit_params)
# 对测量谱进行平滑处理
fit_params, _ = curve_fit(gaussian, range(len(spectrum_norm)), spectrum_norm)
spectrum_smooth = gaussian(range(len(spectrum_norm)), *fit_params)
# 计算光谱响应函数
response = np.divide(np.multiply(reflectance_smooth, light_source_smooth), spectrum_smooth)
return response
```
其中,`light_source` 为光源谱,`reflectance` 为反射谱,`spectrum` 为测量谱。函数通过对这三个谱进行归一化和平滑处理,然后计算光谱响应函数并返回。在代码实现中,使用了高斯函数进行平滑处理,使用 `curve_fit` 函数进行拟合。
阅读全文
相关推荐
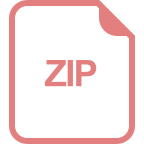
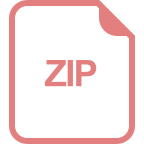
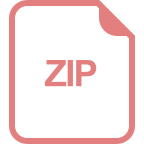
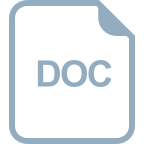
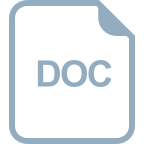
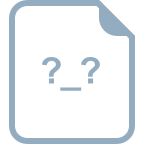
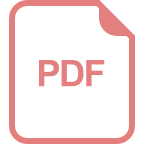
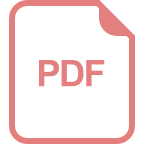
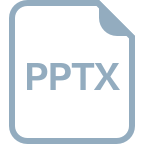
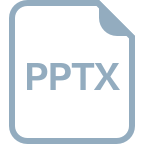
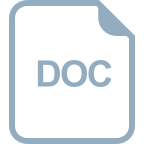
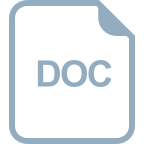
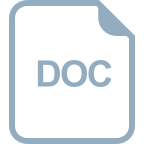
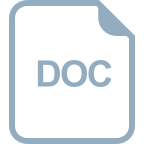
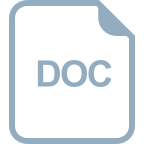
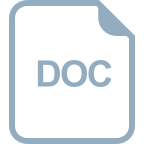
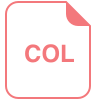
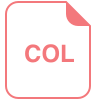
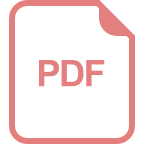