用python将mysql查出的数据导入hbase中
时间: 2024-03-26 20:40:44 浏览: 51
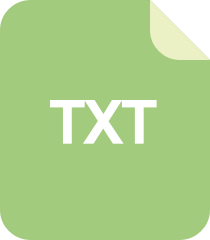
mysql数据导入到hbase中
首先需要安装 `happybase` 和 `pymysql` 两个库,分别用于连接 HBase 和 MySQL 数据库。
安装命令:
```python
pip install happybase pymysql
```
然后,可以使用以下代码将 MySQL 数据库中的数据导入到 HBase 中:
```python
import happybase
import pymysql
# MySQL 数据库连接信息
mysql_host = 'localhost'
mysql_user = 'root'
mysql_password = 'password'
mysql_database = 'database_name'
mysql_table = 'table_name'
# HBase 数据库连接信息
hbase_host = 'localhost'
hbase_table = 'table_name'
# 连接 MySQL 数据库
mysql_conn = pymysql.connect(host=mysql_host, user=mysql_user, password=mysql_password, database=mysql_database)
mysql_cursor = mysql_conn.cursor()
# 连接 HBase 数据库
hbase_conn = happybase.Connection(hbase_host)
hbase_table = hbase_conn.table(hbase_table)
# 查询 MySQL 数据库中的所有数据
mysql_cursor.execute(f"SELECT * FROM {mysql_table}")
results = mysql_cursor.fetchall()
# 遍历所有数据,将数据插入到 HBase 中
for result in results:
row_key = result[0] # 使用 MySQL 数据库中的主键作为 HBase 中的 row key
data = {
'cf:column1': result[1],
'cf:column2': result[2],
# ...
}
hbase_table.put(row_key, data)
# 关闭连接
mysql_cursor.close()
mysql_conn.close()
hbase_conn.close()
```
其中,`cf` 表示列族(column family),`column1` 和 `column2` 表示列名(column name),可以根据实际情况修改。
阅读全文
相关推荐


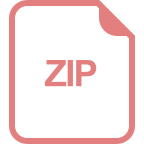
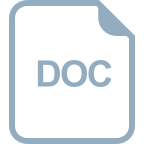

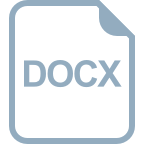
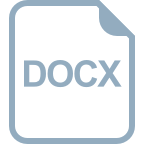





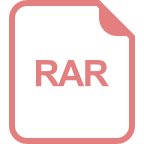
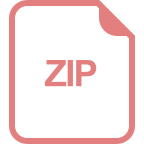
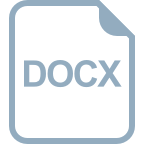