python实现mysql数据导入hbase
时间: 2023-04-27 10:03:19 浏览: 227
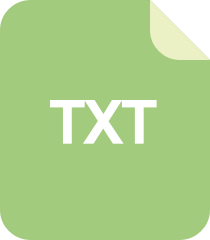
mysql数据导入到hbase中
Python可以通过HBase的Python API来实现将MySQL数据导入HBase的操作。具体步骤如下:
1. 安装HBase的Python API
可以通过pip安装happybase库来使用HBase的Python API:
```
pip install happybase
```
2. 连接HBase
使用happybase库连接HBase:
```python
import happybase
connection = happybase.Connection('localhost')
```
3. 创建HBase表
使用happybase库创建HBase表:
```python
table_name = 'my_table'
column_family = 'cf'
connection.create_table(
table_name,
{column_family: dict()}
)
```
4. 连接MySQL
使用Python的MySQL库连接MySQL:
```python
import mysql.connector
cnx = mysql.connector.connect(
user='user',
password='password',
host='localhost',
database='my_database'
)
```
5. 从MySQL中读取数据
使用MySQL库从MySQL中读取数据:
```python
cursor = cnx.cursor()
query = 'SELECT * FROM my_table'
cursor.execute(query)
for row in cursor:
print(row)
```
6. 将数据导入HBase
使用happybase库将数据导入HBase:
```python
table = connection.table(table_name)
for row in cursor:
key = row[0]
data = {
column_family + ':column1': row[1],
column_family + ':column2': row[2],
column_family + ':column3': row[3]
}
table.put(key, data)
```
完整代码如下:
```python
import happybase
import mysql.connector
connection = happybase.Connection('localhost')
table_name = 'my_table'
column_family = 'cf'
connection.create_table(
table_name,
{column_family: dict()}
)
cnx = mysql.connector.connect(
user='user',
password='password',
host='localhost',
database='my_database'
)
cursor = cnx.cursor()
query = 'SELECT * FROM my_table'
cursor.execute(query)
table = connection.table(table_name)
for row in cursor:
key = row[0]
data = {
column_family + ':column1': row[1],
column_family + ':column2': row[2],
column_family + ':column3': row[3]
}
table.put(key, data)
```
阅读全文
相关推荐




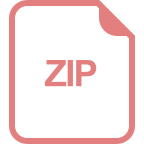
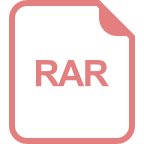
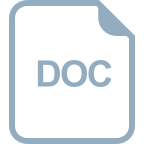
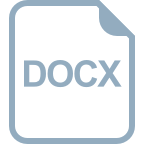
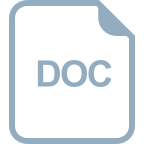
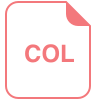
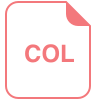
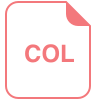
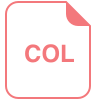
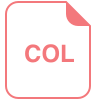

