c++编程设计并实现一个C++联系人类:contact,该类有name,tel和postcode三个私有属性,为该类设计若干方法,包括构造函数、存取三个私有属性的函数、显示联系人信息的函数等。(2)设计并实现一个通讯录类:addressBook,该类包含一个存放所有联系人的容器(可以用实验5中的CArray类实现,也可以用STL的容器实现)。(3)为addressBook设计若干方法,包括显示所有联系人、增加联系人、删除联系人、插入联系人、查找联系人等函数,以及将所有联系人保存到文件和从文件中读取联系人的函数。(4)设计主函数,显示操作选择菜单,完成通讯录的显示、增加、查找、删除和保存等功能5.禁止使用vector
时间: 2023-05-14 21:05:37 浏览: 168
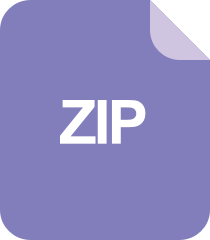
covidsymptom:covidsymptom R软件包提供了来自瑞典COVID症状研究的开放数据。 该软件包包括三个数据集
以下是实现联系人类和通讯录类的代码示例:
```c
#include <iostream>
#include <fstream>
#include <string>
#include <cstring>
#include <cstdlib>
#include <cstdio>
using namespace std;
const int MAX_NAME_LEN = 20;
const int MAX_TEL_LEN = 15;
const int MAX_POSTCODE_LEN = 10;
const int MAX_CONTACTS_NUM = 100;
class Contact {
private:
char name[MAX_NAME_LEN];
char tel[MAX_TEL_LEN];
char postcode[MAX_POSTCODE_LEN];
public:
Contact(const char* n, const char* t, const char* p) {
strncpy(name, n, MAX_NAME_LEN);
strncpy(tel, t, MAX_TEL_LEN);
strncpy(postcode, p, MAX_POSTCODE_LEN);
}
const char* getName() const {
return name;
}
const char* getTel() const {
return tel;
}
const char* getPostcode() const {
return postcode;
}
void setName(const char* n) {
strncpy(name, n, MAX_NAME_LEN);
}
void setTel(const char* t) {
strncpy(tel, t, MAX_TEL_LEN);
}
void setPostcode(const char* p) {
strncpy(postcode, p, MAX_POSTCODE_LEN);
}
void display() const {
cout << "Name: " << name << endl;
cout << "Tel: " << tel << endl;
cout << "Postcode: " << postcode << endl;
}
};
class AddressBook {
private:
Contact* contacts[MAX_CONTACTS_NUM];
int numContacts;
public:
AddressBook() {
numContacts = 0;
for (int i = 0; i < MAX_CONTACTS_NUM; i++) {
contacts[i] = NULL;
}
}
~AddressBook() {
for (int i = 0; i < numContacts; i++) {
delete contacts[i];
}
}
void addContact(Contact* c) {
if (numContacts < MAX_CONTACTS_NUM) {
contacts[numContacts++] = c;
}
}
void deleteContact(int index) {
if (index >= 0 && index < numContacts) {
delete contacts[index];
for (int i = index; i < numContacts - 1; i++) {
contacts[i] = contacts[i + 1];
}
contacts[--numContacts] = NULL;
}
}
void insertContact(int index, Contact* c) {
if (index >= 0 && index < numContacts && numContacts < MAX_CONTACTS_NUM) {
for (int i = numContacts; i > index; i--) {
contacts[i] = contacts[i - 1];
}
contacts[index] = c;
numContacts++;
}
}
Contact* findContact(const char* name) const {
for (int i = 0; i < numContacts; i++) {
if (strcmp(contacts[i]->getName(), name) == 0) {
return contacts[i];
}
}
return NULL;
}
void displayAllContacts() const {
for (int i = 0; i < numContacts; i++) {
contacts[i]->display();
cout << endl;
}
}
void saveToFile(const char* filename) const {
ofstream fout(filename);
if (fout.is_open()) {
fout << numContacts << endl;
for (int i = 0; i < numContacts; i++) {
fout << contacts[i]->getName() << endl;
fout << contacts[i]->getTel() << endl;
fout << contacts[i]->getPostcode() << endl;
}
fout.close();
}
}
void loadFromFile(const char* filename) {
ifstream fin(filename);
if (fin.is_open()) {
int n;
fin >> n;
fin.ignore();
for (int i = 0; i < n; i++) {
char name[MAX_NAME_LEN];
char tel[MAX_TEL_LEN];
char postcode[MAX_POSTCODE_LEN];
fin.getline(name, MAX_NAME_LEN);
fin.getline(tel, MAX_TEL_LEN);
fin.getline(postcode, MAX_POSTCODE_LEN);
Contact* c = new Contact(name, tel, postcode);
addContact(c);
}
fin.close();
}
}
};
int main() {
AddressBook book;
int choice;
do {
cout << "1. Display all contacts" << endl;
cout << "2. Add a contact" << endl;
cout << "3. Find a contact" << endl;
cout << "4. Delete a contact" << endl;
cout << "5. Save to file" << endl;
cout << "6. Load from file" << endl;
cout << "0. Exit" << endl;
cout << "Enter your choice: ";
cin >> choice;
switch (choice) {
case 1:
book.displayAllContacts();
break;
case 2:
char name[MAX_NAME_LEN];
char tel[MAX_TEL_LEN];
char postcode[MAX_POSTCODE_LEN];
cout << "Enter name: ";
cin.ignore();
cin.getline(name, MAX_NAME_LEN);
cout << "Enter tel: ";
cin.getline(tel, MAX_TEL_LEN);
cout << "Enter postcode: ";
cin.getline(postcode, MAX_POSTCODE_LEN);
Contact* c = new Contact(name, tel, postcode);
book.addContact(c);
break;
case 3:
char searchName[MAX_NAME_LEN];
cout << "Enter name to search: ";
cin.ignore();
cin.getline(searchName, MAX_NAME_LEN);
Contact* result = book.findContact(searchName);
if (result != NULL) {
result->display();
}
else {
cout << "Contact not found." << endl;
}
break;
case 4:
int index;
cout << "Enter index to delete: ";
cin >> index;
book.deleteContact(index);
break;
case 5:
char filename[100];
cout << "Enter filename to save: ";
cin.ignore();
cin.getline(filename, 100);
book.saveToFile(filename);
break;
case 6:
char loadFilename[100];
cout << "Enter filename to load: ";
cin.ignore();
cin.getline(loadFilename, 100);
book.loadFromFile(loadFilename);
break;
case 0:
break;
default:
cout << "Invalid choice." << endl;
break;
}
} while (choice != 0);
return 0;
}
```
这个程序实现了一个简单的通讯录,可以添加、删除、查找、显示所有联系人,并支持将联系人保存到文件和从文件中读取联系人。注意,这个程序使用了动态内存分配,需要在析构函数中释放内存。
阅读全文
相关推荐
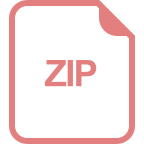
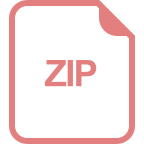



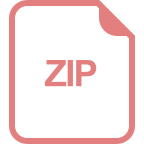
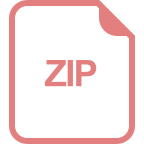
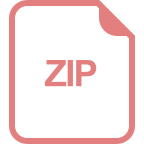
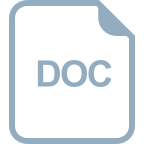
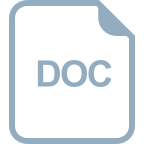
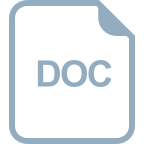






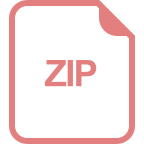