用Python写一段绘制烟花粒子的代码
时间: 2024-01-27 12:05:56 浏览: 29
以下是一段使用 Pygame 库绘制烟花粒子的 Python 代码:
```python
import pygame
import random
pygame.init()
# 设置窗口大小
window = pygame.display.set_mode((800, 600))
# 粒子类
class Particle():
def __init__(self, x, y, radius):
self.x = x
self.y = y
self.radius = radius
self.color = (random.randint(0, 255), random.randint(0, 255), random.randint(0, 255))
self.vx = random.randint(-5, 5)
self.vy = random.randint(-20, -10)
self.gravity = 1
def update(self):
# 更新粒子位置及速度
self.x += self.vx
self.y += self.vy
self.vy += self.gravity
# 绘制粒子
pygame.draw.circle(window, self.color, (int(self.x), int(self.y)), self.radius)
# 移除粒子
if self.y > 600:
particles.remove(self)
# 烟花类
class Firework():
def __init__(self, x, y):
self.x = x
self.y = y
self.exploded = False
self.particles = []
def explode(self):
# 烟花爆炸
for i in range(50):
self.particles.append(Particle(self.x, self.y, random.randint(2, 6)))
self.exploded = True
def update(self):
# 更新烟花状态
if not self.exploded:
pygame.draw.circle(window, (255, 255, 255), (int(self.x), int(self.y)), 4)
if random.randint(1, 60) == 1:
self.explode()
else:
for particle in self.particles:
particle.update()
# 初始化烟花列表
fireworks = []
# 主循环
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
quit()
# 更新烟花状态
for firework in fireworks:
firework.update()
# 添加新烟花
if random.randint(1, 20) == 1:
fireworks.append(Firework(random.randint(0, 800), 600))
# 刷新窗口
pygame.display.update()
window.fill((0, 0, 0))
```
运行代码后,将会在窗口中绘制出烟花效果。每隔一段时间会随机生成一个新的烟花,当烟花到达一定高度时,会爆炸并产生多个彩色粒子。粒子会在空中飞行一段时间后消失。
相关推荐
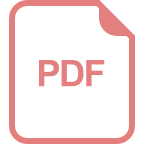
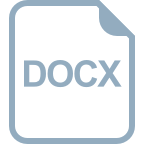
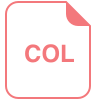
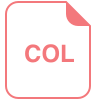
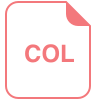
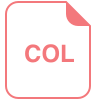
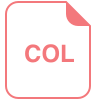









