定义一个“日期”类,含有“年”“月”“日”三个成员变量,含有以下成员函数。 (1) 输入年月日。保证月为1~12,日要符合相应范围。否则报错。 (2)用“年-月-日”的形式打印日期 (3)用“年/月/日”的形式打印日期 (4)比较该日期是否在另一个日期的前面。
时间: 2023-06-18 19:07:17 浏览: 88
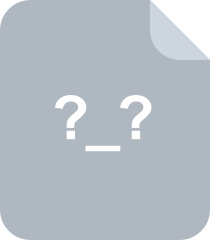
定义一个名为Date的类,用于输入并验证日期

以下是一个基本的“日期”类的实现,包含了题目中要求的成员函数:
```cpp
#include <iostream>
#include <string>
using namespace std;
class Date {
public:
Date(int year, int month, int day) {
if (month < 1 || month > 12 || day < 1 || day > daysInMonth[month - 1]) {
throw invalid_argument("Invalid date");
}
year_ = year;
month_ = month;
day_ = day;
}
string toString() const {
return to_string(year_) + "-" + twoDigits(month_) + "-" + twoDigits(day_);
}
string toSlashString() const {
return to_string(year_) + "/" + twoDigits(month_) + "/" + twoDigits(day_);
}
bool isBefore(const Date& other) const {
if (year_ < other.year_) {
return true;
} else if (year_ > other.year_) {
return false;
} else {
if (month_ < other.month_) {
return true;
} else if (month_ > other.month_) {
return false;
} else {
return day_ < other.day_;
}
}
}
private:
int year_;
int month_;
int day_;
static const int daysInMonth[];
string twoDigits(int n) const {
if (n < 10) {
return "0" + to_string(n);
} else {
return to_string(n);
}
}
};
const int Date::daysInMonth[] = {31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31};
```
在这个实现中,我们通过一个 `Date` 类来表示一个日期。它含有三个私有成员变量 `year_`、`month_` 和 `day_`,分别表示年、月、日。构造函数中会进行参数检查,如果日期不合法则抛出 `invalid_argument` 异常。
成员函数 `toString()` 和 `toSlashString()` 可以将日期按照 “年-月-日” 和 “年/月/日” 的格式转换成字符串。这里使用了 `to_string()` 函数和 `twoDigits()` 函数,后者用于将数字转换成两位的字符串。
最后,成员函数 `isBefore()` 用于比较当前日期是否在另一个日期的前面。比较过程中,我们先比较年份,如果年份相同再比较月份,最后比较日期。如果当前日期在另一个日期的前面,则返回 `true`,否则返回 `false`。
下面是一个示例程序,演示了如何使用 `Date` 类:
```cpp
int main() {
try {
Date d1(2021, 3, 15);
cout << d1.toString() << endl; // 输出:2021-03-15
cout << d1.toSlashString() << endl; // 输出:2021/03/15
Date d2(2021, 3, 16);
cout << d1.isBefore(d2) << endl; // 输出:true
Date d3(2021, 3, 15);
cout << d1.isBefore(d3) << endl; // 输出:false
} catch (const invalid_argument& e) {
cerr << "Error: " << e.what() << endl;
return 1;
}
return 0;
}
```
阅读全文
相关推荐
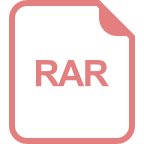
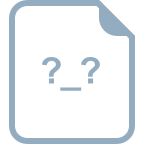
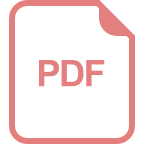
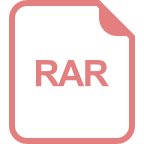
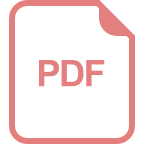
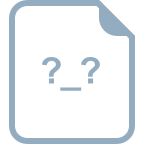
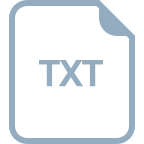
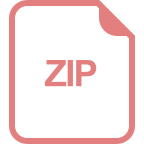
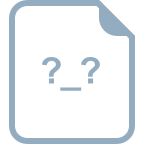
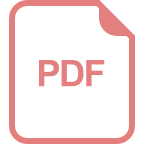
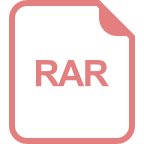
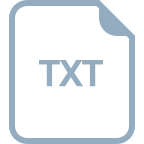
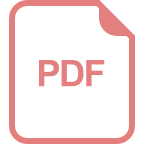
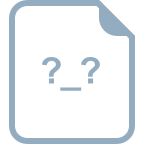
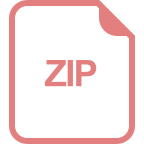
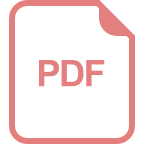
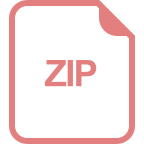
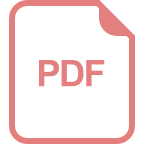