window c++两个进程之间 如何通过消息队列传递消息
时间: 2024-01-27 13:03:30 浏览: 35
在 Windows C++ 中,两个进程之间可以使用消息队列来传递消息。以下是简单的步骤:
1. 创建一个消息队列,可以使用函数 `CreateMessageQueue()`。
2. 在发送进程中,使用函数 `SendMessage()` 将消息发送到消息队列中。
3. 在接收进程中,使用函数 `ReceiveMessage()` 从消息队列中接收消息。
具体实现可以参考以下代码示例:
发送进程:
```c++
#include <windows.h>
#include <iostream>
int main()
{
// 创建一个消息队列
HANDLE hMsgQueue = CreateMailslot(TEXT("\\\\.\\mailslot\\test_mailslot"), 0, MAILSLOT_WAIT_FOREVER, NULL);
if (hMsgQueue == INVALID_HANDLE_VALUE)
{
std::cout << "CreateMailslot failed with error " << GetLastError() << std::endl;
return 1;
}
// 发送消息到消息队列
std::string message = "Hello from sender!";
if (!SendMessage(hMsgQueue, message.c_str(), message.length(), NULL, NULL))
{
std::cout << "SendMessage failed with error " << GetLastError() << std::endl;
CloseHandle(hMsgQueue);
return 1;
}
CloseHandle(hMsgQueue);
return 0;
}
```
接收进程:
```c++
#include <windows.h>
#include <iostream>
int main()
{
// 打开消息队列
HANDLE hMsgQueue = CreateFile(TEXT("\\\\.\\mailslot\\test_mailslot"), GENERIC_READ, FILE_SHARE_READ, NULL, OPEN_EXISTING, FILE_ATTRIBUTE_NORMAL, NULL);
if (hMsgQueue == INVALID_HANDLE_VALUE)
{
std::cout << "CreateFile failed with error " << GetLastError() << std::endl;
return 1;
}
// 从消息队列中接收消息
char buffer[1024];
DWORD bytesRead;
if (!ReadFile(hMsgQueue, buffer, sizeof(buffer), &bytesRead, NULL))
{
std::cout << "ReadFile failed with error " << GetLastError() << std::endl;
CloseHandle(hMsgQueue);
return 1;
}
std::string message(buffer, bytesRead);
std::cout << "Received message: " << message << std::endl;
CloseHandle(hMsgQueue);
return 0;
}
```
注意,这里使用的是邮槽(Mailslot)来实现消息队列,邮槽是 Windows 中一种用于进程间通信的机制。邮槽是一种命名的管道,它可以用于在本地计算机上发送和接收消息。在这里,我们使用邮槽作为消息队列来传递消息。
相关推荐
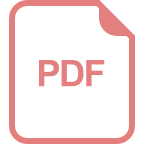














