option60 功能是干什么的
时间: 2023-11-01 14:06:38 浏览: 185
Option 60是DHCP协议中的一个选项,它是用来标识客户端的类型的。当DHCP客户端请求IP地址时,它会发送一个包含Option 60的DHCP请求报文,服务器可以根据Option 60中的值来判断客户端类型,进而提供不同的服务。在某些情况下,Option 60的值还可以用来唯一标识客户端的身份,以便服务器提供更为精细的服务。例如,它可以用来标识VOIP电话或者网络打印机等设备。
相关问题
%option是用来干嘛的
`%option` 是 Flex 中的一个指令,用于设置 Flex 的选项。它可以用来控制 Flex 的一些行为,例如设置 Flex 的输入和输出文件、定义关键字的行为、设置 Flex 的内存分配方式等等。
`%option` 可以接受多个参数,例如:
- `%option outfile="output.c"`:指定输出文件为 `output.c`。
- `%option noyywrap`:禁用 Flex 自带的 yywrap 函数。
- `%option yylineno`:开启行号记录功能。
使用 `%option` 可以让开发者更加灵活地定制 Flex 的行为,以满足不同的需求。
一、功能要求: (1)用文件保存试题库。(每个试题包括题干、4个备选答案、标准答案) (2)试题录入:可随时增加试题到试题库中 (3)试题抽取:每次从试题库中可以随机抽出N道题(N由键盘输入) (4)答题:用户可实现输入自己的答案 (5)自动判卷:系统可根据用户答案与标准答案的对比实现判卷并给出成绩。 二、其它要求: (1)只能使用C语言,源程序要有适当的注释,使程序容易阅读 (2)至少采用文本菜单界面 (3)学生可自动增加新功能模块(视情况可另外加分)
这是一道典型的 C 语言综合应用题,需要实现试题库的文件保存、试题录入、试题抽取、答题和自动判卷等功能。
1. 文件保存试题库:
可以使用文件来保存试题库,每个试题包括题干、4个备选答案、标准答案。可以使用以下结构体来定义试题:
```
typedef struct {
char question[100]; // 题干
char options[4][50]; // 备选答案
int answer; // 标准答案
} Question;
```
可以使用二进制文件来保存试题库,使用 `fwrite()` 函数写入试题,使用 `fread()` 函数读出试题。例如:
```
Question q;
FILE *fp = fopen("questions.dat", "ab");
strcpy(q.question, "What is the capital of France?");
strcpy(q.options[0], "A. Paris");
strcpy(q.options[1], "B. Berlin");
strcpy(q.options[2], "C. London");
strcpy(q.options[3], "D. Rome");
q.answer = 0;
fwrite(&q, sizeof(Question), 1, fp);
fclose(fp);
```
2. 试题录入:
可以使用文件来保存试题库,可以在程序中实现试题录入功能,将试题写入文件中。例如:
```
int add_question() {
Question q;
FILE *fp = fopen("questions.dat", "ab");
printf("Enter the question: ");
fgets(q.question, 100, stdin);
printf("Enter option A: ");
fgets(q.options[0], 50, stdin);
printf("Enter option B: ");
fgets(q.options[1], 50, stdin);
printf("Enter option C: ");
fgets(q.options[2], 50, stdin);
printf("Enter option D: ");
fgets(q.options[3], 50, stdin);
printf("Enter the answer (0-3): ");
scanf("%d", &q.answer);
fwrite(&q, sizeof(Question), 1, fp);
fclose(fp);
return 0;
}
```
3. 试题抽取:
可以从文件中随机读取 N 道试题,可以使用 `srand()` 和 `rand()` 函数结合时间戳来生成随机数,使用 `fseek()` 函数将文件指针移动到相应位置,使用 `fread()` 函数读出试题。例如:
```
int extract_questions(int n) {
Question q;
FILE *fp = fopen("questions.dat", "rb");
int count = 0;
if (fp == NULL) {
printf("Error: cannot open the question file.\n");
return -1;
}
fseek(fp, 0, SEEK_END); // 移动到文件末尾,获取文件大小
long size = ftell(fp);
int num_questions = size / sizeof(Question); // 计算试题数量
srand(time(NULL)); // 设置随机种子
for (int i = 0; i < n; i++) {
int pos = rand() % num_questions; // 随机获取试题位置
fseek(fp, pos * sizeof(Question), SEEK_SET); // 移动到相应位置
fread(&q, sizeof(Question), 1, fp); // 读出试题
printf("Question %d: %s", i+1, q.question);
printf("A. %s", q.options[0]);
printf("B. %s", q.options[1]);
printf("C. %s", q.options[2]);
printf("D. %s", q.options[3]);
count++;
}
fclose(fp);
return count;
}
```
4. 答题:
可以让用户输入自己的答案,可以使用 `scanf()` 函数来实现。例如:
```
int answer_questions(int n) {
Question q;
FILE *fp = fopen("questions.dat", "rb");
int score = 0;
if (fp == NULL) {
printf("Error: cannot open the question file.\n");
return -1;
}
for (int i = 0; i < n; i++) {
fread(&q, sizeof(Question), 1, fp); // 读出试题
printf("Question %d: %s", i+1, q.question);
printf("A. %s", q.options[0]);
printf("B. %s", q.options[1]);
printf("C. %s", q.options[2]);
printf("D. %s", q.options[3]);
int answer;
printf("Enter your answer (0-3): ");
scanf("%d", &answer);
if (answer == q.answer) {
score++;
}
}
fclose(fp);
printf("Your score is %d.\n", score);
return score;
}
```
5. 自动判卷:
可以根据用户答案与标准答案的对比实现判卷并给出成绩。例如:
```
int grade_exam(int n) {
Question q;
FILE *fp = fopen("questions.dat", "rb");
int score = 0;
if (fp == NULL) {
printf("Error: cannot open the question file.\n");
return -1;
}
for (int i = 0; i < n; i++) {
fread(&q, sizeof(Question), 1, fp); // 读出试题
if (q.answer == -1) { // 如果没有标准答案,跳过
continue;
}
printf("Question %d: %s", i+1, q.question);
printf("A. %s", q.options[0]);
printf("B. %s", q.options[1]);
printf("C. %s", q.options[2]);
printf("D. %s", q.options[3]);
printf("The standard answer is: %c\n", 'A'+q.answer);
int answer;
printf("Enter your answer (0-3): ");
scanf("%d", &answer);
if (answer == q.answer) {
score++;
}
}
fclose(fp);
printf("Your score is %d.\n", score);
return score;
}
```
可以使用文本菜单界面来实现各个功能模块之间的切换,例如:
```
int main() {
int choice;
int n;
while (1) {
printf("Please select an option:\n");
printf("1. Add a new question\n");
printf("2. Extract questions\n");
printf("3. Answer questions\n");
printf("4. Grade the exam\n");
printf("5. Quit\n");
printf("Your choice: ");
scanf("%d", &choice);
switch (choice) {
case 1:
add_question();
break;
case 2:
printf("Enter the number of questions to extract: ");
scanf("%d", &n);
extract_questions(n);
break;
case 3:
printf("Enter the number of questions to answer: ");
scanf("%d", &n);
answer_questions(n);
break;
case 4:
printf("Enter the number of questions to grade: ");
scanf("%d", &n);
grade_exam(n);
break;
case 5:
return 0;
default:
printf("Invalid choice.\n");
break;
}
}
return 0;
}
```
以上是一个简单的试题库程序的实现,可以根据实际需求进行修改和完善。
阅读全文
相关推荐
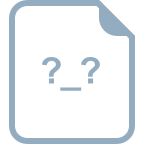
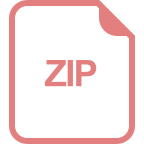
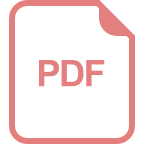






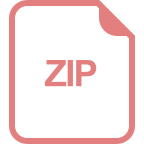
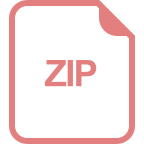
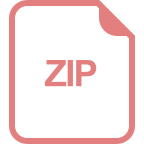
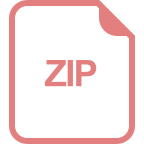
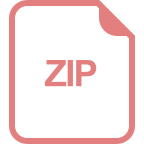