ffmpeg推送H265编码后的裸流,c或者c++代码
时间: 2023-07-28 09:42:34 浏览: 114
您可以使用FFmpeg提供的API将H.265编码后的裸流推送到网络。以下是一个简单的C++示例代码,可以将H.265编码后的视频流推送到RTSP服务器。
```cpp
#include <iostream>
#include <cstdlib>
#include <cstring>
#include <cstdio>
extern "C"
{
#include <libavutil/opt.h>
#include <libavcodec/avcodec.h>
#include <libavformat/avformat.h>
#include <libswscale/swscale.h>
#include <libavutil/imgutils.h>
}
#define STREAM_URL "rtsp://127.0.0.1:8554/live/test"
int main(int argc, char* argv[])
{
// Register all codecs and formats
av_register_all();
avcodec_register_all();
avformat_network_init();
// Open output URL
AVOutputFormat *fmt = av_guess_format("rtsp", NULL, NULL);
AVFormatContext *ofmt_ctx = NULL;
avformat_alloc_output_context2(&ofmt_ctx, fmt, "rtsp", STREAM_URL);
if (!ofmt_ctx) {
std::cerr << "Could not create output context" << std::endl;
return -1;
}
// Add video stream
AVStream *video_stream = avformat_new_stream(ofmt_ctx, NULL);
if (!video_stream) {
std::cerr << "Failed to create video stream" << std::endl;
return -1;
}
video_stream->time_base = (AVRational){1, 25}; // Set FPS
AVCodec *codec = avcodec_find_encoder_by_name("libx265");
AVCodecContext *codec_ctx = avcodec_alloc_context3(codec);
codec_ctx->codec_id = codec->id;
codec_ctx->codec_type = AVMEDIA_TYPE_VIDEO;
codec_ctx->width = 1920;
codec_ctx->height = 1080;
codec_ctx->pix_fmt = AV_PIX_FMT_YUV420P;
codec_ctx->bit_rate = 10000000; // Set bitrate
codec_ctx->rc_min_rate = 5000000; // Set minimum bitrate
codec_ctx->rc_max_rate = 15000000; // Set maximum bitrate
codec_ctx->gop_size = 50; // Set GOP size
codec_ctx->max_b_frames = 0; // Disable B-frames
codec_ctx->time_base = video_stream->time_base;
av_opt_set(codec_ctx->priv_data, "preset", "ultrafast", 0); // Set encoding preset
avcodec_open2(codec_ctx, codec, NULL);
avcodec_parameters_from_context(video_stream->codecpar, codec_ctx);
// Open output stream
if (!(fmt->flags & AVFMT_NOFILE)) {
if (avio_open(&ofmt_ctx->pb, STREAM_URL, AVIO_FLAG_WRITE) < 0) {
std::cerr << "Could not open output URL" << std::endl;
return -1;
}
}
// Write header
if (avformat_write_header(ofmt_ctx, NULL) < 0) {
std::cerr << "Error occurred when opening output URL" << std::endl;
return -1;
}
// Encode and send frame
AVFrame *frame = av_frame_alloc();
uint8_t *frame_buf = (uint8_t*)av_malloc(av_image_get_buffer_size(codec_ctx->pix_fmt, codec_ctx->width, codec_ctx->height, 1));
av_image_fill_arrays(frame->data, frame->linesize, frame_buf, codec_ctx->pix_fmt, codec_ctx->width, codec_ctx->height, 1);
int frame_count = 0;
while (frame_count < 1000) { // Send 1000 frames
av_init_packet(&frame->pkt);
frame->pts = frame_count * video_stream->time_base.den / video_stream->time_base.num;
frame->format = codec_ctx->pix_fmt;
frame->width = codec_ctx->width;
frame->height = codec_ctx->height;
avcodec_send_frame(codec_ctx, frame);
int ret = avcodec_receive_packet(codec_ctx, &frame->pkt);
if (ret >= 0) {
av_interleaved_write_frame(ofmt_ctx, &frame->pkt);
av_packet_unref(&frame->pkt);
}
frame_count++;
}
// Write trailer
av_write_trailer(ofmt_ctx);
// Free resources
avcodec_close(codec_ctx);
avcodec_free_context(&codec_ctx);
avformat_free_context(ofmt_ctx);
av_frame_free(&frame);
av_free(frame_buf);
return 0;
}
```
请注意,这只是一个简单的示例代码,可能需要根据您的需求进行修改。
相关推荐
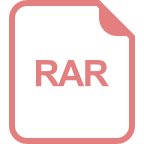
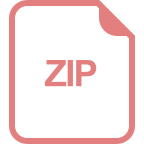














