ffmpeg推送mpp编码后的MppPacket包,c或者c++代码
时间: 2024-03-16 11:42:50 浏览: 142
以下是使用FFmpeg推送MPP编码的MppPacket包的C++代码示例:
```c++
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <stdint.h>
#include <unistd.h>
#include <signal.h>
#include <sys/time.h>
#include <pthread.h>
#include <semaphore.h>
#include <errno.h>
#include <iostream>
#include <string>
// FFmpeg头文件
extern "C" {
#include <libavformat/avformat.h>
#include <libavcodec/avcodec.h>
#include <libswscale/swscale.h>
#include <libavutil/opt.h>
}
using namespace std;
// MPPPacket包结构体
typedef struct {
void* data;
size_t length;
} MppPacket;
// FFmpeg相关变量
AVFormatContext* pFormatCtx;
AVOutputFormat* fmt;
AVStream* video_st;
AVCodecContext* pCodecCtx;
AVCodec* pCodec;
uint8_t* picture_buf;
AVFrame* picture;
int picture_size;
int framecnt=0;
int videoindex=-1;
int in_width = 1280;
int in_height = 720;
int out_width = 1280;
int out_height = 720;
int fps = 25;
// 线程同步用的信号量
sem_t sem;
// FFmpeg推送线程
void* push_thread(void* arg)
{
int ret, got_packet;
AVPacket pkt;
MppPacket* mpp_pkt;
while (true) {
// 等待信号量通知
sem_wait(&sem);
// 从队列中获取待推送的MPPPacket包
// 这里假设是从队列中取出一个MppPacket结构体,包含了MPP编码的数据和数据长度
// 省略队列操作,直接假设mpp_pkt是已经存在的
mpp_pkt = (MppPacket*)malloc(sizeof(MppPacket));
mpp_pkt->data = (void*)malloc(mpp_pkt->length);
// 这里省略将MPP编码数据拷贝到mpp_pkt->data中的操作
// 也可以使用指针指向MPP编码数据的地址
// 将MPPPacket包转换成AVPacket包
av_init_packet(&pkt);
pkt.data = (uint8_t*)mpp_pkt->data;
pkt.size = mpp_pkt->length;
// 发送AVPacket包
ret = avcodec_encode_video2(pCodecCtx, &pkt, picture, &got_packet);
if (ret < 0) {
printf("Error in encoding video frame.\n");
continue;
}
if (got_packet) {
printf("Succeed to encode frame: %5d\tsize:%5d\n", framecnt, pkt.size);
framecnt++;
// 将AVPacket包写入文件或推送到服务器等操作
// 这里省略具体操作,直接释放AVPacket包
av_packet_unref(&pkt);
}
free(mpp_pkt->data);
free(mpp_pkt);
}
return NULL;
}
int main(int argc, char* argv[])
{
int ret;
AVFrame* pFrameYUV;
AVPacket packet;
int y_size;
// 初始化FFmpeg
av_register_all();
avformat_alloc_output_context2(&pFormatCtx, NULL, "flv", NULL);
fmt = pFormatCtx->oformat;
// 打开输出文件(或服务器地址)
ret = avio_open(&pFormatCtx->pb, "rtmp://xxx.xxx.xxx.xxx:1935/live/test", AVIO_FLAG_WRITE);
if (ret < 0) {
printf("Could not open output URL '%s'", "rtmp://xxx.xxx.xxx.xxx:1935/live/test");
return -1;
}
// 添加视频流
video_st = avformat_new_stream(pFormatCtx, 0);
if (video_st == NULL) {
return -1;
}
video_st->time_base.num = 1;
video_st->time_base.den = fps;
// 查找编码器
pCodec = avcodec_find_encoder(AV_CODEC_ID_H264);
if (pCodec == NULL) {
printf("Codec not found.\n");
return -1;
}
// 初始化编码器上下文
pCodecCtx = avcodec_alloc_context3(pCodec);
pCodecCtx->pix_fmt = PIX_FMT_YUV420P;
pCodecCtx->width = out_width;
pCodecCtx->height = out_height;
pCodecCtx->time_base.num = 1;
pCodecCtx->time_base.den = fps;
pCodecCtx->bit_rate = 400000;
// 打开编码器
if (avcodec_open2(pCodecCtx, pCodec, NULL) < 0) {
printf("Could not open codec.\n");
return -1;
}
// 分配AVFrame结构体
picture = av_frame_alloc();
picture_buf = (uint8_t*)av_malloc(avpicture_get_size(pCodecCtx->pix_fmt, pCodecCtx->width, pCodecCtx->height));
avpicture_fill((AVPicture*)picture, picture_buf, pCodecCtx->pix_fmt, pCodecCtx->width, pCodecCtx->height);
// 分配AVFrame结构体
pFrameYUV = av_frame_alloc();
uint8_t* buffer = (uint8_t*)av_malloc(avpicture_get_size(PIX_FMT_YUV420P, pCodecCtx->width, pCodecCtx->height));
avpicture_fill((AVPicture*)pFrameYUV, buffer, PIX_FMT_YUV420P, pCodecCtx->width, pCodecCtx->height);
// 设置线程同步用的信号量
sem_init(&sem, 0, 0);
// 创建推送线程
pthread_t tid;
pthread_create(&tid, NULL, push_thread, NULL);
// 开始推送流
while (true) {
// 这里假设从摄像头或者视频文件中获取一帧YUV数据
// 省略具体操作,直接假设yuv_data是已经存在的
// y_size = in_width * in_height;
// memcpy(picture_buf, yuv_data, y_size * 3 / 2);
// 从摄像头或者视频文件中获取一帧MPPPacket包
// 这里假设从MPP编码器中获取到了一个MppPacket结构体,包含了MPP编码的数据和数据长度
// 省略具体操作,直接假设mpp_pkt是已经存在的
// mpp_pkt = get_mpp_packet();
// 将MPPPacket包送到推送线程中
sem_post(&sem);
// usleep(1000 * 20);
}
// 清理资源
avcodec_close(pCodecCtx);
av_free(pCodecCtx);
av_free(picture_buf);
av_frame_free(&picture);
av_frame_free(&pFrameYUV);
avio_close(pFormatCtx->pb);
avformat_free_context(pFormatCtx);
return 0;
}
```
需要注意的几点:
- 这里假设从摄像头或者视频文件中获取一帧YUV数据,实际使用时需要将这一部分替换成从摄像头或者视频文件中获取YUV数据的代码。
- 这里假设从MPP编码器中获取到了一个MppPacket结构体,包含了MPP编码的数据和数据长度。实际使用时需要将这一部分替换成从MPP编码器中获取MPPPacket包的代码。
- 由于涉及到线程同步,需要使用信号量sem进行同步处理。
- 这里使用了一个线程来推送视频流,需要根据实际情况进行调整。
阅读全文
相关推荐
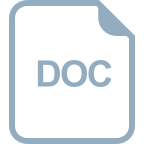
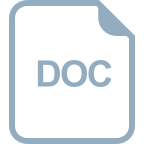
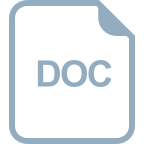
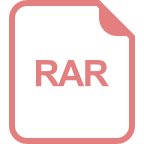

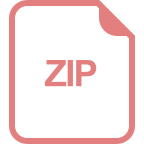
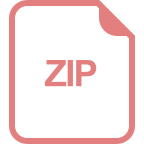
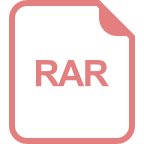
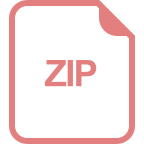
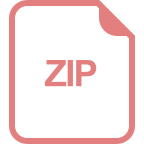
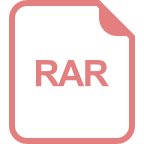
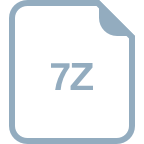
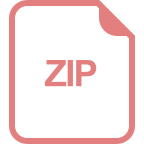